Using HTML
Using HTML in a Suitelet lets you leverage the flexibility of HTML to add elements that are not available in the NetSuite UI components. With HTML you can customize and style your pages in accordance with your organization’s brand and style.
Inline HTML
Use HTML to define a custom field to be included on custom pages generated by Suitelets. For more information, see SuiteScript 2.x Suitelet Script Type.
NetSuite Forms does not support manipulation through the Document Object Model (DOM) and custom scripting in Inline HTML fields. The use of Inline HTML fields on Form pages (except for Forms generated by Suitelets) will be deprecated in a future release.
To add HTML to your Suitelet form, use Form.addField(options) to add a field to the form. Specify the field type as INLINEHTML and use the Field.defaultValue property to set the HTML value.
HTML input is supported only in inline HTML fields.
var htmlImage = form.addField({
id: 'custpage_htmlfield',
type: serverWidget.FieldType.INLINEHTML,
label: 'HTML Image'
});
htmlImage.defaultValue = "<img src='https://<accountID>.app.netsuite.com/images/logos/netsuite-oracle.svg' alt='NetSuite-Oracle logo'>"
SuiteScript does not support direct access to the NetSuite UI through the Document Object Model (DOM). The NetSuite UI should only be accessed using SuiteScript APIs.
HTML Suitelets
HTML Suitelets use HTML elements to build a UI instead of NetSuite UI components. The HTML is embedded in the Suitelet using the https.get(options) method from the N/https Module and the data returned from the form is managed by the Suitelet. Unlike a custom form, which returns prebuilt UI objects, an HTML Suitelet returns a string containing the HTML code, so manipulating and passing data is difficult. Since HTML is coded in a string, passing data to and from the HTML Suitelet requires that you modify the string and change values within the string. The more values you use, the more complex the string manipulation needs to be.
The following example demonstrates a simple volunteer sign-up sheet using an HTML Suitelet. This example can be examined in two parts.
UI Built in HTML
The UI is completely managed by the HTML. It uses standard HTML form elements instead of NetSuite UI components.
The following code sample and screenshot illustrates the form UI built in HTML.
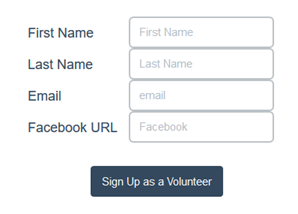
<form method="post" class="form-horizontal" action="https://LinkToSuitelet.js">
<table>
<tbody><tr>
<td>First Name</td>
<td class="col-md-8"><input class="form-control" id="firstname" placeholder="First Name" name="firstname" required="" type="text"></td>
</tr>
<tr>
<td>Last Name</td>
<td class="col-md-8"><input class="form-control" id="lastname" placeholder="Last Name" name="lastname" required="" type="text"></td>
</tr>
<tr>
<td>Email</td>
<td class="col-md-8"><input class="form-control" id="email" placeholder="email" name="email" required="" type="email"></td>
</tr>
<tr>
<td>Facebook URL</td>
<td class="col-md-8"><input class="form-control" id="custentity_fb_url" placeholder="Facebook" name="custentity_fb_url" required="" type="text"></td>
</tr>
<input name="company" value="{{sponsorid}}" type="hidden">
<input name="project" value="{{projectid}}" type="hidden">
</tbody></table>
<br>
<button type="submit" class="btn btn-inverse">Sign Up as a Volunteer</button>
</form>
Form Processing Managed by the Suitelet
The Suitelet manages the information collected from the HTML form. This Suitelet uses the https.get(options) method from the N/https Module to access the content from the HTML page, and uses methods from the N/record Module, N/email Module, and N/search Module to collect, process, and respond to the data the user submits.
/**
*
* @NApiVersion 2.x
* @NScriptType Suitelet
*
*/
define(['N/https', 'N/record', 'N/email', 'N/search'],
function callbackFunction(https, record, email, search) {
function getFunction(context) {
var contentRequest = https.get({
url: "https://LinkToFormPage.html"
});
var contentDocument = contentRequest.body;
var sponsorid = context.request.parameters.sponsorid;
if (sponsorid) {
contentDocument = contentDocument.replace("{{sponsorid}}", sponsorid);
log.debug("Setting Sponsor", sponsorid)
}
var projectid = context.request.parameters.projectid;
if (projectid) {
contentDocument = contentDocument.replace("{{projectid}}", projectid);
log.debug("Setting Project", projectid);
}
context.response.write(contentDocument);
}
function postFunction(context) {
var params = context.request.parameters;
var emailString = "First Name: {{firstname}}\nLast Name: {{lastname}}\nEmail: {{email}}\nFacebook URL: {{custentity_fb_url}}"
var contactRecord = record.create({
type: "contact",
isDynamic: true
});
for (param in params) {
if (param === "company") {
if (params[param] !== "{{sponsorid}}") {
contactRecord.setValue({
fieldId: param,
value: params[param]
});
var lkpfld = search.lookupFields({
type: "vendor",
id: params["company"],
columns: ["entityid"]
});
emailString += "\nSponsor: " + lkpfld.entityid;
}
else {
contactRecord.setValue({
fieldId: "custentity_sv_shn_isindi",
value: true
})
}
}
else {
if (param !== "project") {
contactRecord.setValue({
fieldId: param,
value: params[param]
});
var replacer = "{{" + param + "}}";
emailString = emailString.replace(replacer, params[param]);
}
}
}
var contactId = contactRecord.save({
ignoreMandatoryFields: true,
enableSourcing: true
});
log.debug("Record ID", contactId);
if (params["project"]
["project"] !== "{{projectid}}") {
var lkpfld = search.lookupFields({
type: "job",
id: params["project"],
columns: ["companyname"]
});
emailString += "\nProject Name: " + lkpfld.companyname;
var participationRec = record.create({
type: "customrecord_project_participants",
isDynamic: true
});
participationRec.setValue({
fieldId: "custrecord_participants_volunteer",
value: contactId
})
participationRec.setValue({
fieldId: "custrecord_participants_project",
value: params["project"]
})
var participationId = participationRec.save({
enableSourcing: true,
ignoreMandatoryFields: true
})
}
log.debug("Email String", emailString);
email.send({
author: -5,
recipients: 256,
subject: "New Volunteer Signed Up",
body: "A new volunteer has joined:\n\n" + emailString
});
email.send({
author: -5,
recipients: params["email"],
subject: "Thank you!",
body: "Thank you for volunteering:\n\n" + emailString
});
var contentRequest = https.get({
url: "https://LinkToFormCompletePage.html"
});
var contentDocument = contentRequest.body;
context.response.write(contentDocument);
}
function onRequestFxn(context) {
if (context.request.method === "GET") {
getFunction(context)
}
else {
postFunction(context)
}
}
return {
onRequest: onRequestFxn
};
});
It is possible to simplify the creation of HTML Suitelets through the use of an HTML templating engine. NetSuite servers run FreeMarker, a Java library used to generate text outputs based on templates, to create dynamic pages and forms. For more information about FreeMarker, see http://freemarker.org/docs/index.html