Filter Lines In Your Extensions, Rules, and Constraints
Make sure you filter out lines that you don't want to revise when you create an order management extension or business rule, and that you constrain the changes that you allow on the fulfillment line.
For example:
-
If you create a business rule that modifies a value on a fulfillment line that's still in progress, then filter out lines that are canceled, closed, on backorder, or that Order Management has already sent to billing.
-
Filter out order lines that you already fulfilled. For example, filter out lines that you already shipped for outbound lines or lines that you already received and delivered for return lines.
-
Filter so you only process changes that you make to billing attributes, for example, on the order line's Billing tab, and only if you haven't already sent the order line to billing. For example, modify only the Accounting Rule, Payment Terms, Receivable Transaction Type, and so on. Don't modify any other attributes.
-
Avoid the NullPointerException error. If your logic depends on using an attribute value as part of a calculation, then filter out lines that include an attribute that doesn't contain a value.
-
Filter according to line category code. For example, to process only order lines, not return lines, filter the categoryCode attribute on the fulfillment line according to ORDER. To process only returns, filter it according to the value RETURN.
-
If you use an order management extension, pretransformation rule, or posttransformation rule to set the default values for attributes, then filter out fulfillment lines that reference the original return when you populate the value for the Accounting Rule attribute and Invoicing Rule attribute.
Here are some more details that you might find useful.
-
Filter out lines that aren't shippable. Don't attempt to ship an item that isn't shippable, such as a warranty. For an example that filters out lines that aren't shippable, see Select Fulfillment Lines for Orchestration Process Steps.
-
Filter out lines that reference a coverage item, such as a service agreement. Reserve means you reserve an item in inventory. You don't store a service agreement in inventory because it isn't a physical item, so don't reserve it. For details, see Another Example of Using Extensible Flexfields In Line-Selection Rules.
-
Filter out return lines that you don't want to ship on an outbound sales order. For details, see Prevent Orchestration Process from Shipping Return Lines.
-
Filter out lines that already passed trade compliance. If the line passed, then don't send a request to screen the line for trade compliance. For details, see Use Extensible Flexfields in Line-Selection Rules.
Create Filters In an Order Management Extension
Assume you're revising a line that Order Management is currently fulfilling, so you don't want to revise lines that are closed, canceled, shipped, or that Order Management already sent to accounts receivable. Write an extension that filters the lines.
//
//===========================================================
import oracle.apps.scm.doo.common.extensions.ValidationException;
def lines = header.getAttribute("Lines");
while( lines.hasNext() ) {
def line = lines.next();
Long referenceFlineId = line.getAttribute("ReferenceFulfillmentLineIdentifier");
// If the reference line is null then this isn't a revision.
if(referenceFlineId != null) {
// Get running line if this is a revision.
def runningLine = getLinesFromRunningOrder(referenceFlineId);
if( runningLine == null ) {
// We have an error condition. No fline found with referenceFlineId.
throw new ValidationException("Something's not right. Couldn't find line using reference fline id.");
}
if (runningLine.getAttribute("FulfillLineStatusCode") == "CLOSED" ||
runningLine.getAttribute("FulfillLineCanceledFlag") == "Y" || //Line is cancelled.
runningLine.getAttribute("FulfillLineShippedQty") != null || //Line is shipped.
runningLine.getAttribute("FulfillLineInvoiceInterfacedFlag") == "Y" ){ //Line is interfaced to invoicing.
// This line isn't valid for setting default values.
continue;
}
}
else {
// This sales order doesn't have a revision.
//Its ok to set the default value for attributes.
}
//Put your defaulting logic here.
//line.setAttribute(<attribute name>, <value>);
}
Object getLinesFromRunningOrder(Long runningLineId) {
// Create an instance of the FulfillLinePVO public view object (PVO).
def flinePVO = context.getViewObject("oracle.apps.scm.doo.publicView.analytics.FulfillLinePVO");
// Create a view criteria object.
def vc = flinePVO.createViewCriteria();
// Create a view criteria row.
def vcrow = vc.createViewCriteriaRow();
// Set query conditions on the view criteria row.
vcrow.setAttribute("FulfillLineId", runningLineId);
vc.add(vcrow);
def rowset = flinePVO.findByViewCriteriaWithBindVars(vc, -1, new String [0], new String [0]);
if (rowset.hasNext()) {
def fline = rowset.first();
return fline;
}
}
Create Filters in Business Rules
Write a business rule that filters fulfillment lines, such as in a pretransformation rule, posttransformation rule, line selection criteria, or assignment rule.
Here's an example line selection criteria.
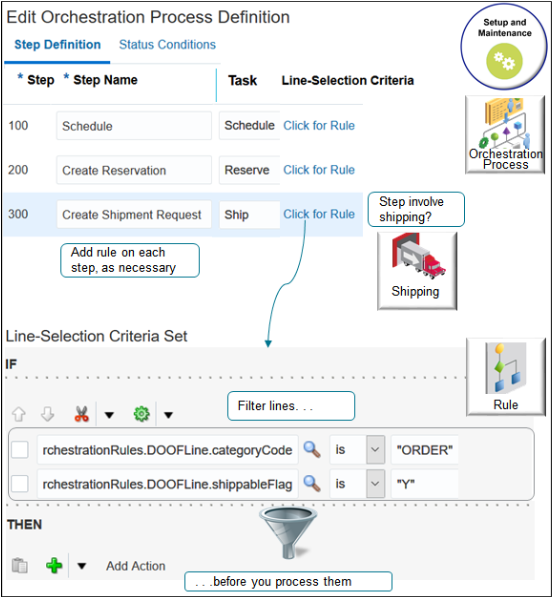
The line selection criteria filters out lines that can't ship so Order Management only sends shippable lines to the fulfillment system that processes shippable lines, such as your shipping system or Oracle Global Order Promising.
Note
-
Use the Manage Orchestration Process Definitions task in the Setup and Maintenance work area.
-
Use the line selection criteria to add the rule.
-
Add the rule to each orchestration process step that shippable affects, as necessary. For example, if the item is a warranty, then it isn't shippable. To filter out the line that isn't shippable, you probably want to add the rule to each step that references a schedule, reserve, or ship task, such as the Schedule step, the Create Reservation step, Create Shipment Request step, and so on.
-
The entire If statement isn't visible in the screen capture. Here are the entire statements:
-
DooSeededOrchestrationRules.DOOFLine.categoryCode is "ORDER"
-
DooSeededOrchestrationRules.DOOFLine.shippableFlag is "Y"
-
-
You can't use Visual Information Builder to select fulfillment lines. You must edit the orchestration process and use Oracle Business Rules.
-
If the nonKitModelFlag attribute contains Y on a line selection criteria, and if the order line contains a configuration model, and if the model contains a kit, then the rule will select the line and process it.
-
Here's the If statement for the rule.
If DooSeededOrchestrationRules.DOOFLine.nonKitModelFlag is Y
-
nonKitModelFlag is a temporary attribute. Its data exists only in active, working memory, so you can't access it later in downstream fulfillment.
-
Example of a Pretransformation Rule
Write a pretransformation rule where you set the value for an attribute, but only after you filter lines. For example:
-
If the order line isn't closed, canceled, shipped, or already sent to billing, then set the default value for the requested ship date attribute.
For more about posttransformation rules, see Overview of Transformation Rules.
Constrain Changes That You Allow on Fulfillment Lines
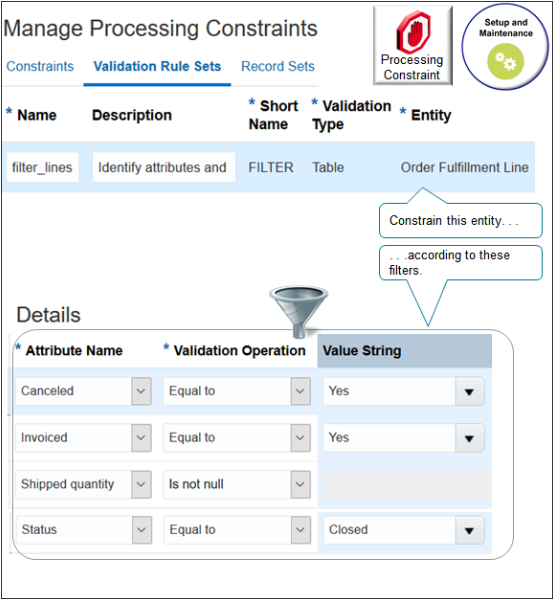
Note
-
Use the Manage Processing Constraints task in the Setup and Maintenance work area.
-
Set the Entity attribute to Order Fulfillment Line.
-
Use the Details area to add your filters.
Assume that if the fulfillment line is closed or canceled, or if Order Management already shipped it or invoiced it, then you don't want your users to modify a value on the line, such as Ship-to Site.
Attribute Name
Validation Operation
Value String
Canceled
Equal To
Yes
Invoiced
Equal To
Yes
Shipped Quantity
Is Not Null
-
Status
Equal To
Closed
You can also create a constraint that prevents you from submitting a sales order that doesn't include payment terms for lines that meet a specific criteria. For example, the line isn't a return line, or the line is for a transfer. For details, see Display Payment Terms on Sales Orders.