3Technical Notes
Technical Notes
This section lists necessary technical information and consist following topics:
Web Services and REST Overview
API Introduction
An Application Programming Interface (API) is a tool used by applications to provide external applications or users to grant access to specific features of the application. Typically, this involves the passing of some argument data to a web URL (also known as an Endpoint) that has access to the API. For example, within Oracle WMS Cloud application there is an API for invoking the input data processing (init stage interface). In order to accomplish this, the API needs to know things like company, facility, the interface name, and other key pieces of information to execute correctly. Our API's allow the application to expose discrete pieces of functionality to other applications or users in a controlled manner without the need to give access to the entire system and without requiring the user interface to be used.
API Request
Each API is given a specific URL hosted as part of the WMS application. The APIs use HTTPS protocol to receive requests and return a response in much the same way that submitting a form on a website works within a browser. When the "form" is submitted, a call to a URL is made over HTTPS, which has the ability to transmit this data within the request. The data can then be extracted from the received request within the WMS application and used to run the API.
Requirements:- Must be of type POST
- The Content-Type should be "application/x-www-form-urlencoded"
- This allows the data to be sent in key-value pairs
- Any non-ASCII data must be URL encoded to ensure data integrity
- See https://www.w3schools.com/tags/ref_urlencode.asp
- Any URL reserved characters (; / ? : @ = &) in the data must also be properly encoded to ensure data integrity
- The key-value pairs are represented in the request in the format myurl.com?key1=value1&key2=value2...
- If the reserved characters are not encoded in the data itself, they can be misunderstood to have special meaning and cause data corruption when parsing the request.
API Response
Once the API has completed (successfully or not) within the WMS application, an HTTP response is sent back to the requester. WMS APIs will always return a response. This is similar in the way in which a webpage is returned to a requesting user's browser. However, instead of webpage data, all Oracle WMS Cloud APIs are designed to give a standardized response.
Web Services and REST Overview
This section is intended to give a high level overview of web services, how they work, and how customers use them. Web services are a common method by which machines are able to passing data, files, or invoking a process over the internet using the HTTP protocol. The two main flavors of web services are SOAP (Simple Object Access Protocol) and REST (Representational State Transfer). This section will focus primarily on REST (a service based on REST is called a RESTful service) as it's the web service method used by Oracle WMS Cloud.
- The main object of web services is to provide a window to a resource on a server
- A resource can be a document, picture, video, web page, API, or anything that can be represented in a computer system
- RESTful services are lightweight, maintainable, and scaleable (all important things for a cloud application)
- HTTP is the underlying protocol used by web services
- This is the same protocol you just used to request this web page (a resource!) in your browser
- HTTP provides mechanisms to handle the requests and responses to RESTful services
- This includes transferring data and/or files
- The Client/Service/Server Relationship
- A client is the system connecting to and making a request to a service hosted on a server
- The server processes the request, returns a response to the client, and closes the connection
- Clients and services talk to each other via messages
- HTTP messages follow a request and response cycle; each request by the client requires a response from the server
- It's possible to not get a response from the server. That typically means there was an issue with the server's execution of the request.
- Requests to a service and responses from a service are both structured messages
- The actual message is just a series of lines of plain text (see Request Example below)
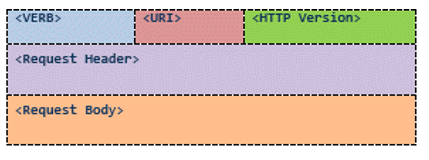
An HTTP request is really nothing more than several lines of text that tell a client about the request it needs to execute.
Here we will discuss the components of a request and then walk through an example using the Google Chrome extension, POSTman.
- Verb
- An HTTP method that defines the action of the request
- Examples: GET, POST, PUT, DELETE, ...
- WMS primarily requires clients to POST to our resources
- Some clients and Jitterbit may utilize GET
- URI (Uniform Resource Identifier)
- A URI is a resource on a server that can be accessed by a service
- The most common form of URI is a URL (Uniform Resource Locator)
- A URL specifies both the primary access mechanism and network location
- In simple terms a URL identifies the network and location of the resource being accessed
- Example: http://example.org/wiki/Main_Page
- This URL refers to the resource /wiki/Main_Page that is obtained via HTTP from a network whose domain name is example.org
- HTTP Version
- Current version is "HTTP v1.1"
- Request Header
- Contains request metadata in a collection of key-value pairs
- In general, this is information about the request, the requesting client, authorization, and the format of any data in the request body
- The most important header keys for WMS's purposes are:
- Authorization - An encrypted username/password combination that may be required to access the service
- Content-Type - Defines the format and possibly the encoding (charset) of the data in the request body for POST requests
- The format is known as a MIME Type
- Important POST MIME Types:
- application/x-www-form-urlencoded
- Alphanumeric data is encoded (convert legal non-ASCII characters to a representation using allowed characters) and sent in key-value pairs in the request body
- Any illegal characters, like ñ, are encoded to an ASCII hex representation like "%XX" and then decoded back after transmission
- Example: If you have one field "Name" with a value of "Mary" and another field "Gender" set to "Male", it would be represented as: Name=Mary&Gender=Male
- multipart/form-data
- The data is sent in key-value pairs in the request body in multiple parts
- Typically used for transmitting files (binary data)
- Good for transmitting large amounts of data
- application/xml
- The content of the request body is XML
- Request Body
- The actual content (data) of the message
- Format (and possibly encoding) is determined by the Content-Type header
- Key-value pairs are represented in the format: key1=value1&key2=value2&key3=value3...
- key/value are separated by "="
- pairs are separated by "&"
- However, if for example the Content-Type is set to "application/xml" there would be no key-value pairs, just an XML message
Oracle WMS Cloud Request Example (key-value pairs)
Using the POSTman Google Chrome extension, here's a sample HTTP request:
- A request verb of POST
- A URI (URL) of http://xxxxxxxxxxxxxxx/dummy_endpoint/test
- An Authorization header (I had put in a username/password and POSTman encrypted it for me)
- A Content-Type header of "application/x-www-form-urlencoded", which tells us that the data in the request body will be key-value pairs:
- Even though you don't see this explicitly in the headers screenshot above, it will be present in the actual request shown below
- 4-data keys with corresponding values in the request body: first_name, last_name, gender, and extra_data
When we convert this request from the POSTman UI to HTTP:
This is the request information that is actually transmitted!
- It tells HTTP that we want to POST a request to the host xxxxxxxxxxxx for resource /dummy_endpoint/test using HTTP version 1.1
- It also shows that we have the request headers Authorization, Cache-Control, Postman-Token, and Content-Type:
- You don't need to worry about Cache-Control or Postman-Token
- It is shows the request body data as key-value pairs represented in the format discussed
- This is expected since the Content-Type is set to "x-www-form-urlencoded"
- Finally, we can see that it encoded the illegal ñ character to "%C3%B1" for transmission
- %C3%B1 is the UTF-8 representation of ñ
Request Example (XML)
<Person> <FirstName>Jane</FirstName> <LastName>Doe</LastName> <Gender>female</Gender> <ExtraData>ñ</ExtraData> </Person>
Using the POSTman Google Chrome extension, the following HTTP request was created:
You can see from the screenshots that we have:
- A request verb of POST
- A URI (URL) of http://xxxxxxxxxxxxxxxxxx/dummy_endpoint/test
- An Authorization header (I had put in a username/password and POSTman encrypted it for me)
- A Content-Type header of "application/xml", which tells us that the data in the request body will be XML
- An XML message in the request body
When we convert this request from the POSTman UI to HTTP:
- It tells HTTP that we want to POST a request to the host xxxxxxxxxxxxxxxx for resource /dummy_endpoint/test using HTTP version 1.1
- It also shows that we have the request headers Authorization, Cache-Control, Postman-Token, and Content-Type:
- You don't need to worry about Cache-Control or Postman-Token
- It is shows the request body XML data:
- The XML has been encoded for transmission since characters like "<" and ">" are illegal for HTTP
Notice that the data is the same as before, just represented in an XML format that was made up for this example.
This is done to show that the same data can be passed via many different methods and formats using web services.
What's most important is that the two communicating system agree on these details up front so that each system knows what to expect.
How to Get Started with Oracle WMS Cloud Web Services
This section describes the basic steps necessary to get setup so as to use Oracle WMS Cloud Web Services:
- Login to a Cloud WMS environment with an ADMIN Role user
- Open the Group Configuration screen and create a group with no UI or RF menus
- In the entry field at the top start typing in Group
- Select the group, click the permissions button and in the drilldown screen, select can_run_ws_stage_interface permission and save it
- Open the users screen and copy your user (using the duplicate button which is the button next to the one with the plus sign on the right) and make the following change before saving it:
- Change the Login
- Change the Role from ADMIN to Employee
- Enter a Password and note it down
- Change the employee number
- Change the first and last name
- Use Postman to create a request using the technical notes section and try to post using this new userid and password
- Use asynch=True so that you will get any functional validation errors back
- Open the relevant screen (such as Purchase Order if you're uploading PO's) to check if it loaded
- If not, open the input interface screen and select purchase order to see if there are any errors listed