When buyers go through the normal checkout process, instead of clicking the Place order button on the order confirmation screen, they can click the Create scheduled order button. They are then directed to the Scheduled Order page (user/scheduled_order_new.jsp
), where they can specify the name and type (daily, weekly or monthly) of the scheduled order.
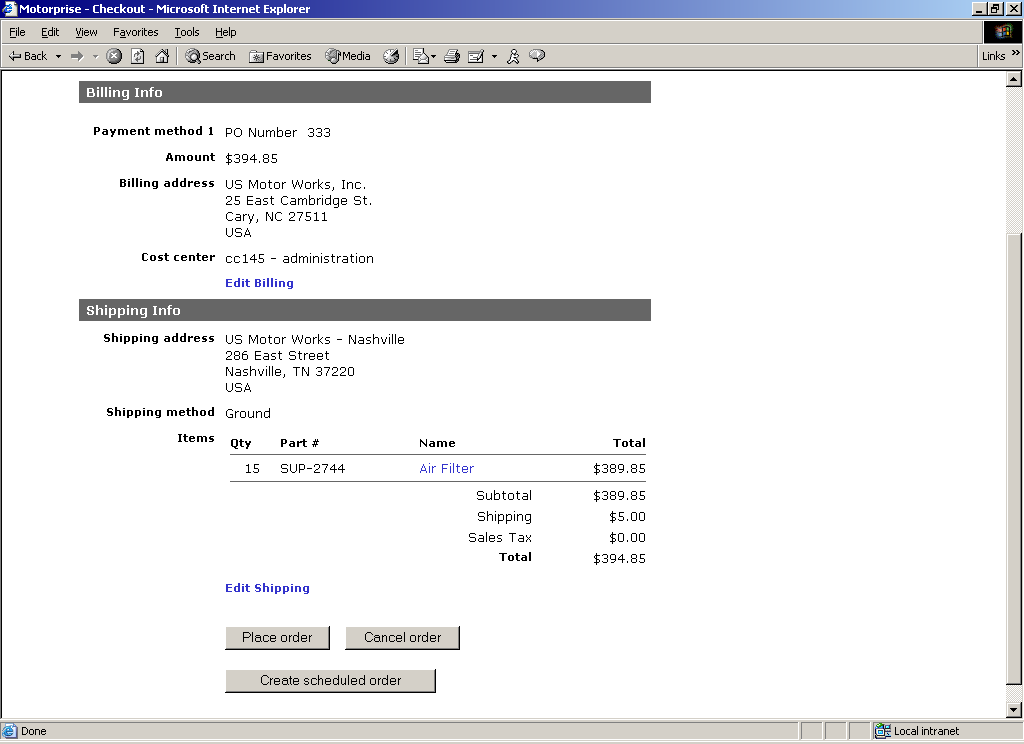
Scheduling an order during checkout.
The following is the code in scheduled_order_new.jsp
used to create a scheduled order.
First we find the ID number for the order by checking if prototypeOrderId
is set or not:
<dsp:droplet name="IsNull">
<dsp:param name="value" param="prototypeOrderId"/>
<dsp:oparam name="true">
Then we use the ScheduledOrderFormHandler
to let the user fill in the order information:
<tr valign=bottom> <td valign="top" align=right><span class=smallb>Name</span></td> <td><dsp:input bean="ScheduledOrderFormHandler.value.name" name="name" size="35" type="text"/><br> <span class=help>Enter a name for your scheduled order (i.e. Weekly Spark Plug Assortment).</span></td> </tr> <tr> <td align=right><span class=smallb>Schedule type</span></td> <td align="left"> <dsp:select bean="ScheduledOrderFormHandler.moveToMode" name="select" size="1"> <dsp:option value=""/>Choose schedule <dsp:option value="Daily"/>daily <dsp:option value="Weekly"/>weekly <dsp:option value="Monthly"/>monthly </dsp:select> </td> </tr> <tr> <td align="right" valign=top><span class=smallb>Order</span></td> <td> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. templateOrderId.userInputFields.orderId"> <dsp:droplet name="ForEach"> <dsp:param name="array" param="result"/> <dsp:oparam name="outputStart"> <dsp:option value=""/> Select a fulfilled order </dsp:oparam> <dsp:oparam name="output"> <dsp:getvalueof id="elementId" idtype="String" param="element.id"> <dsp:option value="<%=elementId%>"/><dsp:valueof param="element.id"/> </dsp:getvalueof> </dsp:oparam> </dsp:droplet> </dsp:select> <br> </td> </tr> <tr><td><img src="../images/d.gif"></td></tr> <tr> <td></td> <input name="createNew" type="hidden" value="<dsp:valueof param="createNew"/>"> <dsp:input bean="ScheduledOrderFormHandler.moveToErrorURL" type="hidden" value="scheduled_order_new.jsp"/> <dsp:input bean="ScheduledOrderFormHandler.moveToSuccessURL" type="hidden" value="scheduled_order_calendar.jsp?createNew=new"/> <td><dsp:input bean="ScheduledOrderFormHandler.moveToURL" type="submit" value="Continue"/></td> </tr>
We used the same page, scheduled_order_new.jsp
, to create scheduled orders from the checkout page and from My Account. (If the user creates a new scheduled order from the Scheduled Orders page in My Account, we display a list of all the user’s fulfilled orders. See the Scheduling Orders in My Account section below for more information.) Based on the hidden form field prototypeOrderId
, which is set in the order confirmation screen, we determine whether we are creating a scheduled order from the checkout process.
When the user chooses to create a scheduled order from the order confirmation page, we assign the ID of the order being created to prototypeOrderId
and pass it to scheduled_order_new.jsp
, which assigns the order ID to ScheduledOrderFormHandler.complexScheduledOrderMap.
.
templateOrderId.userInputFields.orderId
Once the buyer enters the name of the order and the schedule type, he or she is directed to scheduled_order_calendar.jsp
, which displays a calendar for the order depending on its schedule type: daily, weekly or monthly. We used the following JSP code to display the appropriate calendar:
<dsp:form action="scheduled_order_preview.jsp" method="post"> <table border=0 cellpadding=8> <tr valign=bottom> <td align=right><span class=smallb>Order name</span></td> <td><dsp:valueof bean="ScheduledOrderFormHandler.value.name" /></td> </tr> <tr> <td align=right><span class=smallb>Schedule type</span></td> <td> <dsp:valueof bean="ScheduledOrderFormHandler.moveToMode" /> scheduled order</td> </tr> <dsp:droplet name="Switch"> <dsp:param bean="ScheduledOrderFormHandler.moveToMode" name="value"/> <dsp:oparam name="Daily"> <tr> <td align="right"><span class=smallb>Order placement</span></td> <td align="left"> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.scheduleMode" name="select" size="1"> <dsp:option value="onceDaily"/>once a day. <dsp:option value="twiceDaily"/>twice a day. </dsp:select> </td> </tr> <%@ include file="day_of_week.jspf" %> </dsp:oparam> <dsp:oparam name="Weekly"> <dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.scheduleMode" type="hidden" value="Weekly"/> <%@ include file="day_of_week1.jspf"%> <tr> <td align="right" valign="top"><span class=smallb>Week(s) of month</span></td> <td align="left"> <table border="0"> <tr> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="1"/>1st. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="2"/>2nd. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="3"/>3rd. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="Checkbox" value="4"/>4th. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="5"/>5th. </td> </tr> <tr><td colspan=5><span class=help>Uncheck to ship selected weeks</span></td></tr> </table> </tr> </dsp:oparam> <dsp:oparam name="Monthly"> <tr> <td align="right"><span class=smallb>Order Placement</span></td> <td align="left"> <dsp:select bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. scheduleMode" name="select" size="1"> <dsp:option value="onceMonthly"/>once a month. <dsp:option value="biMonthly"/>every two months. <dsp:option value="quarterly"/>every quarter. </dsp:select> </td> </tr> </dsp:oparam> <dsp:oparam name="default"> </dsp:oparam> </dsp:droplet>
The above block of code shows the control flow we used to display the appropriate calendar chosen by the user and set in ScheduleOrderFormHandler.moveToMode
. There are three schedule types: daily, weekly, and monthly. The calendar for each schedule type is explained below.
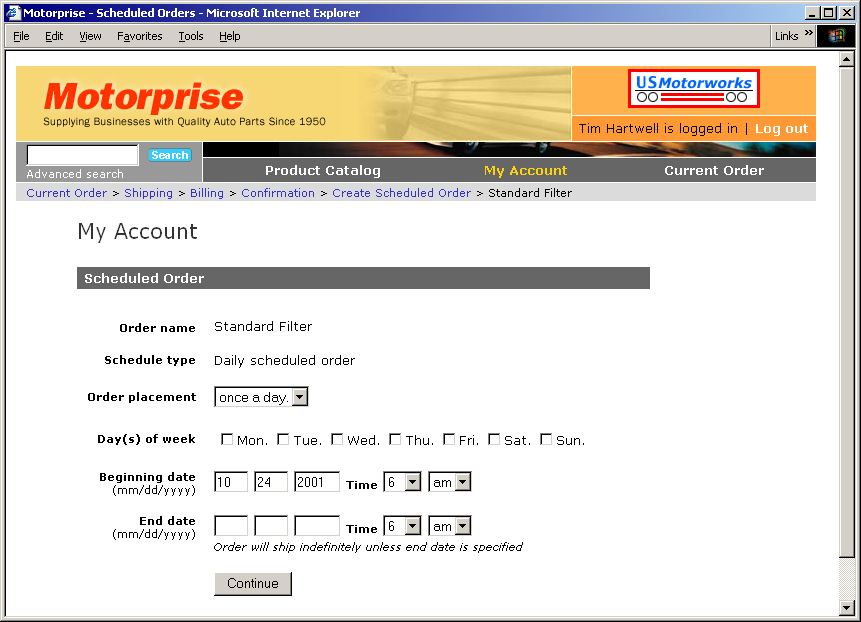
Calendar page for creating a daily scheduled order.
The code for Daily schedule is:
<dsp:oparam name="Daily"> <tr> <td align="right"><span class=smallb>Order placement</span></td> <td align="left"> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.scheduleMode" name="select" size="1"> <dsp:option value="onceDaily"/>once a day. <dsp:option value="twiceDaily"/>twice a day. </dsp:select> </td> </tr> <%@ include file="day_of_week.jspf" %> </dsp:oparam>
The day_of_week.jspf
page fragment contains the following code that displays the days of the week and assigns them to complexScheduledOrderMap
as shown below:
dsp:importbean bean="/atg/commerce/order/scheduled/ScheduledOrderFormHandler"/> <!-- Selection of days for shipment including x times/week --> <tr> <td align="right"><span class=smallb>Day(s) of week</span></td> <td align="left"> <table> <tr> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.daysOfWeek" name="daysOfWeek" type="checkbox" value="2"/>Mon. </td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.daysOfWeek" name="daysOfWeek" type="checkbox" value="3"/>Tue. </td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.daysOfWeek" name="daysOfWeek" type="checkbox" value="4"/>Wed. </td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.daysOfWeek" name="daysOfWeek" type="Checkbox" value="5"/>Thu. </td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.daysOfWeek" name="daysOfWeek" type="checkbox" value="6"/>Fri. </td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.daysOfWeek" name="daysOfWeek" type="Checkbox" value="7"/>Sat. </td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.daysOfWeek" name="daysOfWeek" type="Checkbox" value="1"/>Sun. </td> </tr> </table> </tr> </dsp:page>
As shown above, we display the options for Daily schedule, once a day and twice a day, and the days of the week with corresponding checkboxes from which to choose.
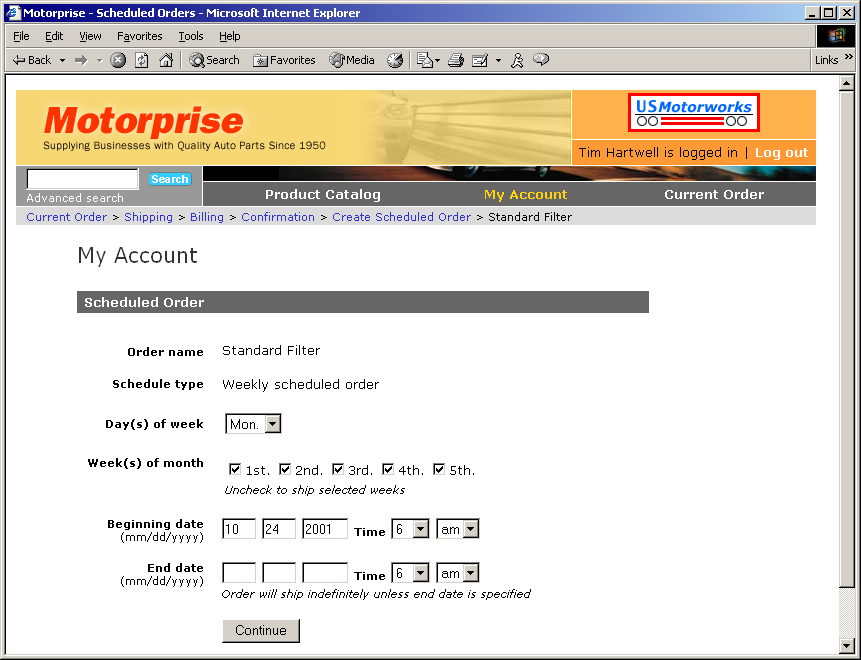
Calendar page for creating a weekly scheduled order.
The code for Weekly schedule is:
<dsp:oparam name="Weekly"> <dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. calendarSchedule.userInputFields.scheduleMode" type="hidden" value="Weekly"/> <%@ include file="day_of_week1.jspf"%> <tr> <td align="right" valign="top"><span class=smallb>Week(s) of month</span></td> <td align="left"> <table border="0"> <tr> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="1"/>1st. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="2"/>2nd. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="3"/>3rd. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="Checkbox" value="4"/>4th. </td> <td><dsp:input bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. occuranceInMonth" name="occuranceInMonth" type="checkbox" value="5"/>5th. </td> </tr> <tr><td colspan=5><span class=help>Uncheck to ship selected weeks</span></td></tr> </table> </tr> </dsp:oparam>
The code above sets the ScheduledOrderFormHandler.scheduledMode
to Weekly and displays the days of the week in a select box for the user to choose any day of the week. It also displays all the weeks in the month numerically and provides corresponding checkboxes so the user can choose a week to schedule the order.
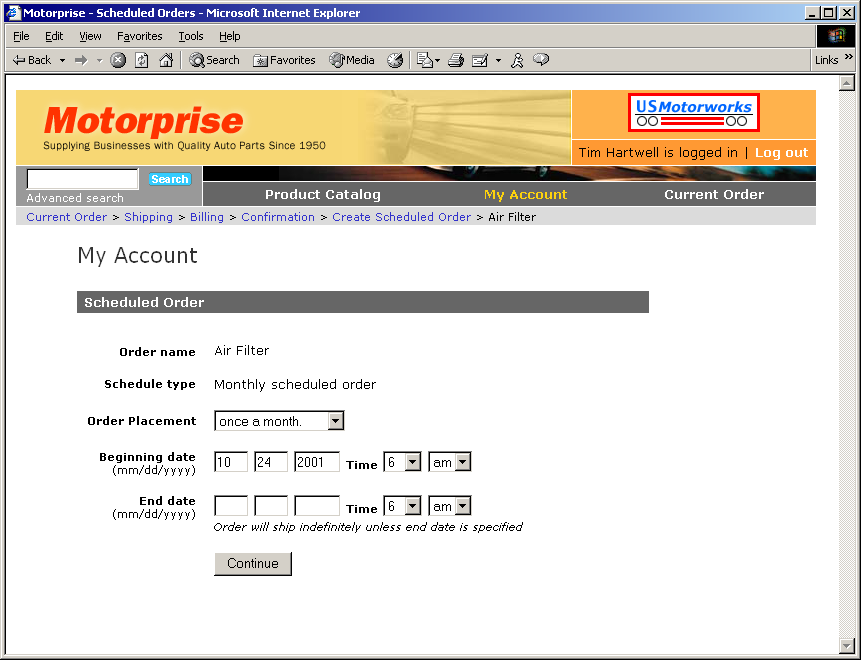
Calendar page for creating a monthly scheduled order.
The following is the code to display Monthly schedule:
<dsp:oparam name="Monthly"> <tr> <td align="right"><span class=smallb>Order Placement</span></td> <td align="left"> <dsp:select bean="ScheduledOrderFormHandler. complexScheduledOrderMap.calendarSchedule.userInputFields. scheduleMode" name="select" size="1"> <dsp:option value="onceMonthly"/>once a month. <dsp:option value="biMonthly"/>every two months. <dsp:option value="quarterly"/>every quarter. </dsp:select> </td> </tr> </dsp:oparam>
There are three modes for the Monthly schedule from which the user can choose: once a month, every two months, every quarter.
Finally, for each calendar, we display fields for start and end dates. The following code snippet is from start_end_date.jspf
, which is invoked in scheduled_order_new.jsp
, to display the start and end date options:
<tr valign=top> <td align=right><span class=smallb>Beginning date</span><br> <span class="small">(mm/dd/yyyy)</span></td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. startDate.userInputFields.month" maxlength="2" size="2" type="text"/> <dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. startDate.userInputFields.day" maxlength="2" size="2" type="text"/> <dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. startDate.userInputFields.year" maxlength="4" size="4" type="text"/> <span class=smallb>Time</span> <dsp:droplet name="Switch"> <dsp:param bean="Profile.locale" name="value"/> <dsp:oparam name="en_US"> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. startDate.userInputFields.hour"> <dsp:droplet name="For"> <dsp:param name="howMany" value="12"/> <dsp:oparam name="output"> <dsp:getvalueof id="numHoursEn" idtype="Integer" param="count"> <dsp:option value="<%=numHoursEn.toString()%>"/> <dsp:valueof param="count"/> </dsp:getvalueof> </dsp:oparam> </dsp:droplet> </dsp:select> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. startDate.userInputFields.ampm"> <dsp:option value="am"/> am <dsp:option value="pm"/> pm </dsp:select> </dsp:oparam> <dsp:oparam name="de_DE"> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. startDate.userInputFields.hour"> <dsp:droplet name="For"> <dsp:param name="howMany" value="24"/> <dsp:oparam name="output"> <dsp:getvalueof id="numHoursDe" idtype="Integer" param="count"> <dsp:option value="<%= numHoursDe.toString() %>"/> <dsp:valueof param="count"/> </dsp:getvalueof> </dsp:oparam> </dsp:droplet> </dsp:select> </dsp:oparam> </dsp:droplet> </td> </tr> <tr valign=top> <!--<dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. endDate.userInputFields.mode" type="hidden" value="definite"/>--> <td align=right><span class=smallb>End date</span><br> <span class="small">(mm/dd/yyyy)</span></td> <td><dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. endDate.userInputFields.month" maxlength="2" size="2" type="text"/> <dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. endDate.userInputFields.day" maxlength="2" size="2" type="text"/> <dsp:input bean="ScheduledOrderFormHandler.complexScheduledOrderMap. endDate.userInputFields.year" maxlength="4" size="4" type="text"/> <span class=smallb>Time</span> <dsp:droplet name="Switch"> <dsp:param bean="Profile.locale" name="value"/> <dsp:oparam name="en_US"> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. endDate.userInputFields.hour"> <dsp:droplet name="For"> <dsp:param name="howMany" value="12"/> <dsp:oparam name="output"> <dsp:getvalueof id="hourEndDe" idtype="Integer" param="count"> <dsp:option value="<%=hourEndDe.toString()%>"/> <dsp:valueof param="count"/> </dsp:getvalueof> </dsp:oparam> </dsp:droplet> </dsp:select> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap.endDate. userInputFields.ampm"> <dsp:option value="am"/> am <dsp:option value="pm"/> pm </dsp:select> </dsp:oparam> <dsp:oparam name="de_DE"> <dsp:select bean="ScheduledOrderFormHandler.complexScheduledOrderMap. endDate.userInputFields.hour"> <dsp:droplet name="For"> <dsp:param name="howMany" value="24"/> <dsp:oparam name="output"> <dsp:getvalueof id="hourEndEn" idtype="Integer" param="count"> <dsp:option value="<%=hourEndEn.toString()%>"/> <dsp:valueof param="count"/> </dsp:getvalueof> </dsp:oparam> </dsp:droplet> </dsp:select> </dsp:oparam> </dsp:droplet>
We display submit buttons so that user can choose any of the options provided above and preview the order. The following is the relevant code from scheduled_order_new.jsp
:
<tr> <td></td> <input name="createNew" type="hidden" value="<dsp:valueof param="createNew"/>"> <dsp:input bean="ScheduledOrderFormHandler.moveToErrorURL" type="hidden" value="scheduled_order_new.jsp"/> <dsp:input bean="ScheduledOrderFormHandler.moveToSuccessURL" type="hidden" value="scheduled_order_calendar.jsp?createNew=new"/> <td><dsp:input bean="ScheduledOrderFormHandler.moveToURL" type="submit" value="Continue"/></td> </tr>
Once the user chooses the calendar options and clicks the Continue button, he or she is directed to scheduled_order_preview.jsp
, which displays the order information and a submit button to create the scheduled order.
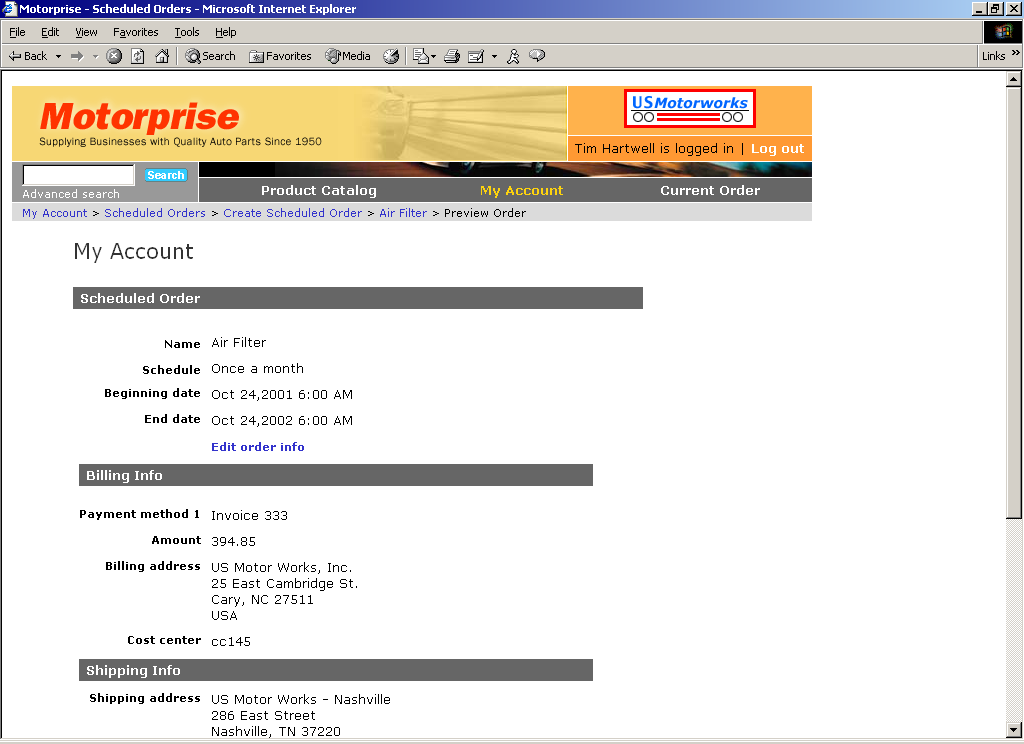
Previewing the scheduled order.
The following is the code from scheduled_order_preview.jsp
for displaying the submit button for creating or deleting the scheduled order:
<dsp:droplet name="IsNull"> <dsp:param name="value" bean="ScheduledOrderFormHandler.repositoryId"/> <dsp:oparam name="true"> <dsp:input bean="ScheduledOrderFormHandler.createErrorURL" type="hidden" value="scheduled_order_new.jsp"/> <dsp:input bean="ScheduledOrderFormHandler.createSuccessURL" type="hidden" value="scheduled_orders.jsp"/> <dsp:input bean="ScheduledOrderFormHandler.create" type="submit" value="Create scheduled order"/> </dsp:oparam> <dsp:oparam name="false"> <dsp:input bean="ScheduledOrderFormHandler.deleteSuccessURL" type="hidden" value="scheduled_orders.jsp"/> <dsp:input bean="ScheduledOrderFormHandler.deleteErrorURL" type="hidden" value="scheduled_order_preview.jsp"/> <dsp:input bean="ScheduledOrderFormHandler.delete" type="submit" value="Delete scheduled order"/><p> <span class=smallb><dsp:a href="scheduled_orders.jsp">Return to scheduled orders</dsp:a></span> </dsp:oparam> </dsp:droplet>
The user is then directed to scheduled_orders.jsp
, which actually displays all his or her scheduled orders. The following is the code used to fetch and display the scheduled orders for a given user:
<dsp:droplet name="RQLQueryForEach"> <dsp:param bean="/atg/dynamo/transaction/TransactionManager" name="transactionManager"/> <dsp:param bean="/atg/commerce/order/OrderRepository" name="repository"/> <dsp:param name="itemDescriptor" value="scheduledOrder"/> <dsp:param name="queryRQL" value="profileId=:profileId"/> <dsp:param bean="Profile.repositoryId" name="profileId"/> <dsp:oparam name="output"> <dsp:a href="scheduled_order_preview.jsp"> <dsp:param name="scheduledOrderId" param="element.repositoryId"/> <dsp:param name="source" value="scheduledOrder"/> <dsp:valueof param="element.name"/> </dsp:a><br> </dsp:oparam> <dsp:oparam name="empty"> You have no scheduled orders. </dsp:oparam> </dsp:droplet>
We used the RQLQueryForEach
servlet bean to fetch the scheduled orders for a given user and provide a link to scheduled_order_preview.jsp
for each order. It assigns the repository ID of each scheduled order to the scheduledOrderId
parameter and passes it to the page. For more information on the RQLQUeryForEach
servlet bean, please refer to the ATG Page Developer’s Guide.