SuiteScript 2.x User Event Script Tutorial
A user event script is a server script that is triggered by actions taken on a record. The script type can include logic that is executed in three situations:
-
After the user has started the process of opening the record but before the record loads.
-
After the user clicks Save to save the record.
-
After the record is submitted to the database.
This tutorial walks you through the implementation of a basic user event script. It includes the following sections:
-
Step One: Check Your Prerequisites
-
Step Two: Create the Script File
-
Step Three: Review the Script (Optional)
-
Step Four: Upload the Script File to NetSuite
-
Step Five: Create a Script Record and Script Deployment Record
-
Step Six: Test the Script
Before proceeding, review SuiteScript 2.x Script Basics for an explanation of terms used in this tutorial. For an overview of the basic structural elements required in any SuiteScript 2.x entry point script, see SuiteScript 2.x Anatomy of a Script.
Sample Script Overview
The sample script is meant to be deployed on the employee record. When it is, it takes the following actions:
-
When a user begins the process of creating a new employee record, the script disables the Notes field before the page loads.
-
After the user enters all required information about the employee and clicks Save, the script adds a value to the Notes field that states that no date has yet been set for the new employee’s orientation.
-
After the record has been submitted, the script creates a task record for scheduling the new employee’s orientation session. The script assigns the task to the new employee’s supervisor.
The script uses all three of the entry points available with the SuiteScript 2.x User Event Script Type. For details about these entry points, see beforeLoad(context), beforeSubmit(context), and afterSubmit(context).
Check Your Prerequisites
To complete this tutorial, your system has to be set up properly. Review the following before you proceed:
Enable the Feature
Before you can complete the rest of the steps listed in this topic, the Client and Server SuiteScript features must be enabled in your account. For help enabling these features, see Enabling SuiteScript.
Create an Employee Record
To complete this tutorial, your system must have an employee record that you can designate as the supervisor of a new test employee.
To view your existing employee records, select Lists > Employees. If your account does not already have an employee record you can use, create one. For help creating an employee record, see the help topic Adding an Employee.
Create the Script File
Before proceeding, you must create the entry point script file. To create the file, copy and paste the following code into the text editor of your choice. Save the file and name it createTask.js.
/**
*@NApiVersion 2.x
*@NScriptType UserEventScript
*/
// Load two standard modules.
define ( ['N/record', 'N/ui/serverWidget'] ,
// Add the callback function.
function(record, serverWidget) {
// In the beforeLoad function, disable the Notes field.
function myBeforeLoad (context) {
if (context.type !== context.UserEventType.CREATE)
return;
var form = context.form;
var notesField = form.getField({
id: 'comments'
});
notesField.updateDisplayType({
displayType: serverWidget.FieldDisplayType.DISABLED
});
}
// In the beforeSubmit function, add test to the Notes field.
function myBeforeSubmit(context) {
if (context.type !== context.UserEventType.CREATE)
return;
var newEmployeeRecord = context.newRecord;
newEmployeeRecord.setValue({
fieldId: 'comments',
value: 'Orientation date TBD.'
});
}
// In the afterSubmit function, begin creating a task record.
function myAfterSubmit(context) {
if (context.type !== context.UserEventType.CREATE)
return;
var newEmployeeRecord = context.newRecord;
var newEmployeeFirstName = newEmployeeRecord.getValue ({
fieldId: 'firstname'
});
var newEmployeeLastName = newEmployeeRecord.getValue ({
fieldId: 'lastname'
});
var newEmployeeSupervisor = newEmployeeRecord.getValue ({
fieldId: 'supervisor'
});
if (newEmployeeSupervisor) {
var newTask = record.create({
type: record.Type.TASK,
isDynamic: true
});
newTask.setValue({
fieldId: 'title',
value: 'Schedule orientation session for ' +
newEmployeeFirstName + ' ' + newEmployeeLastName
});
newTask.setValue({
fieldId: 'assigned',
value: newEmployeeSupervisor
});
try {
var newTaskId = newTask.save();
log.debug({
title: 'Task record created successfully',
details: 'New task record ID: ' + newTaskId
});
} catch (e) {
log.error({
title: e.name,
details: e.message
});
}
}
}
// Add the return statement that identifies the entry point funtions.
return {
beforeLoad: myBeforeLoad,
beforeSubmit: myBeforeSubmit,
afterSubmit: myAfterSubmit
};
});
Review the Script (Optional)
If you want to understand more about how this script is structured, review the following subsections.
JSDoc Tags
The following image shows the JSDoc block used in this sample script. For an explanation of the numbered callouts, see the table that follows the image.
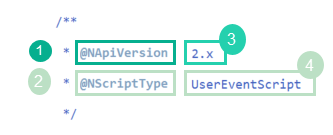
Callout |
Description |
---|---|
1 and 3 |
The @NApiVersion tag and its value (2.x). This tag is required in all entry point scripts. Valid values are 2.0, 2.x, and 2.X. |
2 and 4 |
The @NScriptType tag and its value (UserEventScript). This tag identifies the script type being used. The value is not case sensitive, but using Pascal case, as shown in this example, allows the script to be more easily read. |
beforeLoad Function
The following image shows the myBeforeLoad function. Because of the way the script’s return Statement is structured, this function executes when the beforeLoad entry point is invoked.

Callout |
Description |
---|---|
1 |
The |
2 |
This statement tells the system that the rest of the logic in the function should execute only if the user is creating a new record. The statement uses the |
3 |
These statements tell the system to disable the Notes field. They do so in part by using the |
beforeSubmit Function
The following image shows the myBeforeSubmit function. Because of the way the script’s return Statement is structured, this function executes when the beforeSubmit entry point is invoked.
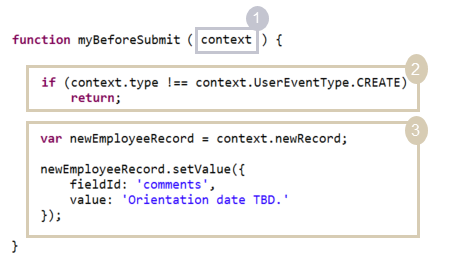
Callout |
Description |
---|---|
1 |
The Note that the properties available to this context object are different from those available to the beforeLoad Function, which is linked to the beforeLoad entry point. The beforeLoad entry point has access to a form property, but the beforeSubmit object does not, which means that the beforeSubmit entry point function does not have access to the form used by the record. |
2 |
This statement tells the system that the rest of the logic in the function should execute only if the user is creating a new record. The statement uses the |
3 |
These statements add text to the Notes field of the new record. They do so by using the |
afterSubmit Function
The following image show the myAfterSubmit function. Because of the way the script’s return Statement is structured, this function executes when the afterSubmit entry point is invoked.
The first part of the function retrieves data from the employee record:
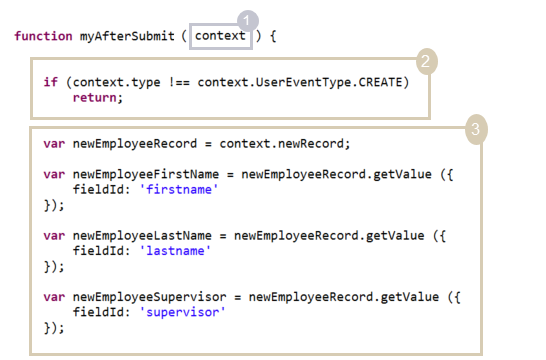
Callout |
Description |
---|---|
1 |
The |
2 |
This statement tells the system that the rest of the logic in the function should execute only if the user is creating a new record. The statement uses the |
3 |
These statements retrieve several pieces of data from the record by using the context object’s |
The rest of the function creates the task record:
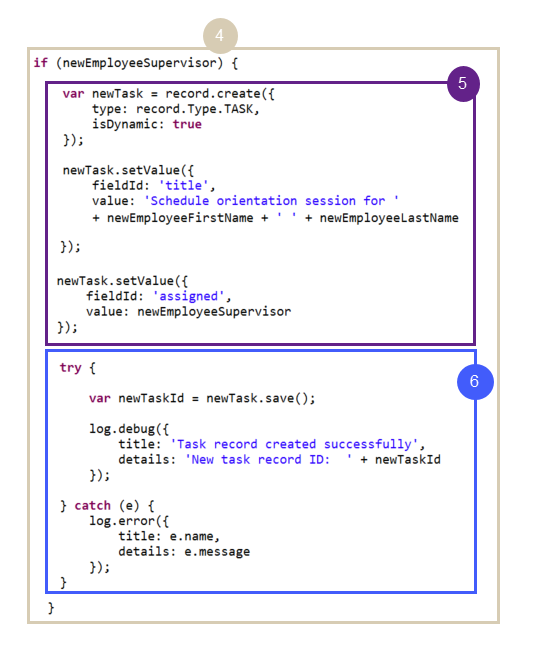
Callout |
Description |
---|---|
4 |
A conditional statement. The expression inside the parentheses evaluates to true if a value has been set for the Supervisor field. If this condition is met, the system executes the logic within the curly braces. |
5 |
These statements use N/record Module APIs to create a task record ( |
6 |
This code attempts to save the new task record. If any errors are encountered, details are written to the script deployment record’s execution log. |
return Statement
The following image shows the callback function’s return statement.
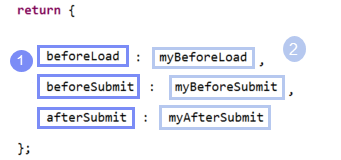
Callout |
Description |
---|---|
1 |
Entry points. The callback function’s return statement must use at least one entry point that belongs to the script type identified by the @NScriptType tag (Callout 4 in JSDoc Tags). This script uses all three of the user event script type’s entry points. |
2 |
Entry points functions. For each entry point used, your script must identify an entry point function that is defined elsewhere within the script. |
Upload the Script File to NetSuite
After you have created your entry point script file, upload it to your NetSuite File Cabinet.
To upload the script file:
-
In the NetSuite UI, go to Documents > Files > SuiteScripts.
-
In the left pane, select the SuiteScripts folder and click Add File.
-
Follow the prompts to locate the createTask.js file (created in Step Two) in your local environment and upload it.
Note that even after you upload the file, you can edit it from within the File Cabinet, if needed. For details, see the help topic Editing Files in the File Cabinet.
Create a Script Record and Script Deployment Record
In general, before an entry point script can execute in your account, you must first create a script record that represents the entry point script file. You must also create a script deployment record.
To create the script record and script deployment record:
-
Go to Customization > Scripting > Scripts > New.
-
In the Script File dropdown list, select createTask.js.
Note that, if you had not yet uploaded the file, as described in Step Four, you could upload the file from this page. Clicking the plus sign icon to the right of the dropdown list opens a window that lets you select and upload a file. You may have to move your cursor over the area to the right of the dropdown list to display the plus sign icon.
-
After you have selected the script file and it is displayed in the dropdown list, click the Create Script Record button.
The system displays a new script record if the script file passes validation checks, and the createTask.js file is listed on the Scripts subtab.
-
Fill out the required body fields as follows:
-
In the Name field, enter Create Task Record User Event.
-
In the ID field, enter _ues_create_task_record.
-
-
Click the Deployments subtab.
-
Add a line to the sublist, as follows:
-
Set the Applies to dropdown list to Employee.
-
In the ID field, enter _ues_create_task_record.
Leave the other fields set to their default values. Note that the Status field is set to Testing, which means that the script does not deploy for other users. (If you wanted to change the deployment later and make the customization’s behavior available to all users, you could edit the deployment and set the status to Released.)
-
-
Click Save.
The system creates the script and script deployment records.
Test the Script
Now that the script is deployed, you should verify that it executes as expected.
To test the script:
-
Begin the process of creating a new employee record by selecting Lists > Employees > Employees > New.
-
In the new employee form, verify that the Notes field is disabled. If the beforeLoad entry point function worked as expected, the field is gray and cannot be edited.
-
Enter value for required fields. These fields may vary depending on the features enabled in your account and any customizations that exist. At a minimum, you must enter values for the following:
-
Name — Enter a first name of John and last name of Smith.
-
Subsidiary — (OneWorld only) Choose an appropriate value from the dropdown list.
-
-
To make sure that the afterSubmit function executes correctly, enter a value in the Supervisor field, if you have not already.
-
Click Save. A success message appears, and the system displays the new record in View mode.
-
Verify that the beforeSubmit function was successful: Look at the Notes field. It should include the value Orientation date TBD as shown below.
-
Verify that the afterSubmit function was successful:
-
Select Activities > Scheduling > Tasks.
-
Check the filtering options to make sure that your view includes tasks assigned to all users.
-
Verify that a task was created and assigned to the person you named as a supervisor in Step 4.
-
Next Steps
If you want to learn how to customize this entry point script so that it calls a custom module, see SuiteScript 2.x Custom Module Tutorial.