2 Overview of the JavaFX WebView Component
This chapter introduces the JavaFX embedded browser, a user interface component that provides a web viewer and full browsing functionality through its API.
The embedded browser component is based on WebKit, an open source web browser engine. It supports Cascading Style Sheets (CSS), JavaScript, Document Object Model (DOM), and HTML5.
The embedded browser enables you to perform the following tasks in your JavaFX applications:
-
Render HTML content from local and remote URLs
-
Obtain Web history
-
Execute JavaScript commands
-
Perform upcalls from JavaScript to JavaFX
-
Manage web pop-up windows
-
Apply effects to the embedded browser
The embedded browser inherits all fields and methods from the Node
class, and therefore, it has all its features.
The classes that constitute the embedded browser reside in the javafx.scene.web
package. Figure 2-1 shows the architecture of the embedded browser and how it relates to other JavaFX classes.
Figure 2-1 Architecture of the Embedded Browser
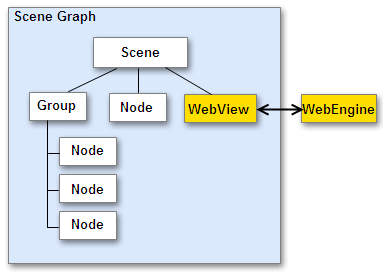
Description of "Figure 2-1 Architecture of the Embedded Browser"
WebEngine Class
The WebEngine
class provides basic web page functionality. It supports user interaction such as navigating links and submitting HTML forms, although it does not interact with users directly. The WebEngine
class handles one web page at a time. It supports the basic browsing features of loading HTML content and accessing the DOM as well as executing JavaScript commands.
Two constructors enable you to create a WebEngine
object: an empty constructor and a constructor with the specified URL. If you instantiate an empty constructor, the URL can be passed to a WebEngine
object through the load
method.
Starting JavaFX SDK 2.2, developers can enable and disable JavaScript calls for a particular web engine and apply custom style sheets. User style sheets replace the default styles on the pages rendered in this WebEngine
instance with user-defined ones.
WebView Class
The WebView
class is an extension of the Node
class. It encapsulates a WebEngine
object, incorporates HTML content into an application's scene, and provides properties and methods to apply effects and transformations. The getEngine()
method called on a WebView
object returns a web engine associated with it.
Example 2-1 shows the typical way to create WebView
and WebEngine
objects in your application.
PopupFeatures Class
The PopupFeatures
class describes the features of a web pop-up window as defined by the JavaScript specification. When you need to open a new browser window in your application, the instances of this class are passed into pop-up handlers registered on a WebEngine
object by using the setCreatePopupHandler
method as shown in Example 2-2.
Example 2-2 Creating a Pop-Up Handler
webEngine.setCreatePopupHandler(new Callback<PopupFeatures, WebEngine>() { @Override public WebEngine call(PopupFeatures config) { // do something // return a web engine for the new browser window } });
If the method returns the web engine of the same WebView
object, the target document is opened in the same browser window. To open the target document in another window, specify the WebEngine
object of another web view. When you need to block the pop-up windows, return the null
value.
Other Features
When working with the WebView
component, you should remember that it has the default in-memory cache. It means that any cached content is lost once the application containing the WebView
component is closed. However, developers can implement cache at the application level by means of the java.net.ResponseCache
class. From WebKit perspectives, the persistent cache is a property of the network layer similar to connection and cookie handlers. Once some of those are installed, the WebView
component uses them in transparent manner.