Predefined Methods
This section describes the predefined methods available per object. These predefined methods can be divided into two categories: methods that are available on all or many objects, called generic methods, and methods that are only available on particular objects, called specific methods.
Generic Methods
This section describes generic methods
getSequenceNumber
Allows retrieval of a unique number from a user defined sequence. Pass the code of the user defined sequence. Note that the sequence must have been configured via the "/usersequences" resource before it can be used.
lookUpInsurableEntity
Looks up the insurable entity in the system based on the input parameters passed.
Availability
This method is available in the dynamic logic (groovy script)
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Code of the object/person |
In |
String |
Usage name of the insurable entity type of the insurable entity |
Out |
Insurable Entity |
Insurable Entity |
lookUpPerson
Looks up the person in the system based on the input parameters passed.
Availability
This method is available in the dynamic logic (groovy script).
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Code of the person |
Out |
Person |
Person |
Please refer to the OHI Claims Adjudication and Pricing Implementation Guide for the list of other available generic methods.
lookUpRelation
Looks up the person or organization in the system based on the input parameters passed.
Availability
This method is available in the dynamic logic (groovy script).
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Code of the relation |
Out |
Relation |
Relation |
lookUpProvider
Looks up the provider in the system based on the input parameters passed.
Availability
This method is available in the dynamic logic (groovy script).
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Code of the provider |
In |
String |
Definition code of the provider |
Out |
provider |
Provider |
lookUp
Looks up the object in the system based on the input parameters passed.
Availability
This method is available in the dynamic logic (groovy script).
Parameters
In / Out | Type | Description |
---|---|---|
In |
Class<?> |
The class of the object that needs to be looked up. |
In |
Map<String,Object> |
A map of key/value pairs to uniquely identify the object. The key of the map should be the attribute name to search on, like 'code' or 'startDate'. The value should be the value that needs to be searched on, so it should be a string for code or a Date for a date type argument. |
Out |
Object |
Object found after performing the search. The lookup will be restricted to provide only the first object, so it is advised to be restrictive in the parameters passed in. |
Example
def premiumSchedule = lookUp(PremiumSchedule.class, ['code': 'PS1'])
Search Methods
Please refer to the OHI Claims Adjudication and Pricing – Dynamic Logic Guide for the list of available search methods
Object Methods
In this section, the methods are described that are only available on specific objects. For each method, the following information is given:
-
The purpose of the method.
-
On which object(s) the method can be called.
-
Description of the parameters.
addAssignedProvider
Adds an assigned provider to a person (for example through a Policy Validation Rule).
Availability
This method is available for the Person object. Its purpose is to have the possibility to add an assigned provider through function dynamic logic.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Provider code. Mandatory |
In |
String |
Provider flex code definition code. Mandatory |
In |
String |
Provider assignment type code. Mandatory |
In |
Date |
Assigned provider start date. Mandatory |
In |
Date |
Assigned provider end date. Optional |
Example
person.addAssignedProvider('1234567890', 'US_PROVIDER', 'PCP', java.sql.Date.valueOf('2017-01-01'), null)
addBrokerAgent
Creates a policyBrokerAgent with the passed in broker, agent and startDate. The newly added policyBrokerAgent is added to the policyBrokerAgent detail list. If the passed in broker or agent does not exist in the system, the dynamic logic using this method fails with a technical error (OHI-DYLO-007).
Availability
This method is available on Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The broker code of the new policyBrokerAgent. |
In |
String |
The agent code of the new policyBrokerAgent. |
In |
Date |
The startDate of the new policyBrokerAgent. Mandatory |
Out |
Policy Broker Agent |
The newly created copy of the policyBrokerAgent |
Example
def newPolicyBrokerAgent = policy.addBrokerAgent("newBROKERA", null, new java.sql.Date(Date.parse("yyyy-MM-dd", "2017-01-01").time))
addContractAlignment
Adds a contract alignment to a person (for example through a Policy Validation Rule).
Availability
This method is available for the Person object. Its purpose is to have the possibility to add a contract alignment through function dynamic logic.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Capitation contract code. Mandatory |
In |
Date |
Contract alignment start date. Mandatory |
In |
Date |
Contract alignment end date. Optional |
Example
person.addContractAlignment('CONTR001', java.sql.Date.valueOf('2017-01-01'), java.sql.Date.valueOf('2017-12-12'))
addGroupClientEvent
Adds an event record to a group client
Availability
This method is available for Group Client. Its purpose is to add an event record to the group client:
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The description of the event. Mandatory |
In |
Datetime |
The date and time the event happend. Mandatory |
In |
String |
The code of the policy that is related to the group client event. Optional |
In |
String |
The code of the group account that is related to the group client event. Optional |
Example
In the below example two dynamic fields on the group client event (user and ext_id) are populated
groupClient.addGroupClientEvent('New Group Account added' , new java.sql.Date(Date.parse("yyyy-MM-dd", "2020-01-01").time) , 'POL1234' , 'GRAC_ABC' )
setGroupClientStatus
Sets the status of a group client.
Availability
This method is available for Group Client. It sets the status to 'CHANGED'. It does not allow setting the status to APPROVED
Parameters
The method has no parameters
Example
groupClient.setgroupClientStatus()
addMessage
Adds a message to a policy or group client (for example through a Policy Validation Rule).
Availability
This method is available for Policy and Group Client. Its purpose is to have the possibility to add a message through function dynamic logic:
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Message code. Mandatory |
In |
Object[] |
Message arguments. Optional |
Example
policy.addMessage('CMPL_01')
groupClient.addMessage('CRCL_01')
addParameterValue
Adds a parameter value to the policyEnrollmentProduct.
Availability
This method is available for PolicyEnrollmentProduct.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Object |
ParameterValue |
Example
policyEnrollmentProduct.addPolicyAddOn("PARAM_01", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time), null, 600, null, null, null, "USD")
addPolicyAddOn
Adds an add-on to the policyEnrollmentProduct.
Availability
This method is available for PolicyEnrollmentProduct.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Object |
PolicyAddOn |
Example
policyEnrollmentProduct.addPolicyAddOn("ADDON_01", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time), null)
addPolicyBillReceiver
Adds a bill receiver to the policyPremiumBillAllocation.
Availability
This method is available for PolicyPremiumBillAllocation.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Relation code. Optional |
In |
BigDecimal |
Percentage. Mandatory |
In |
String |
Billing Account code. Optional |
Example
policyPremiumBillAllocation.addPolicyBillReceiver("REL83746", 50, "")
addPolicyEnrollmentInsurableClass
Adds an insurable class to the policyEnrollment.
Availability
This method is available for PolicyEnrollment.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Insurable Class code. Mandatory |
In |
Date |
The startDate of the new policyEnrollmentInsurableClass. Mandatory |
Example
policyEnrollment.addPolicyEnrollmentInsurableClass("EMPLOYEE", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
addPolicyGroupAccount
Adds a group account to the policy.
Availability
This method is available for Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Group Account code. Mandatory |
In |
Date |
The startDate of the new policyGroupAccount. Mandatory |
Example
policy.addPolicyGroupAccount("ORCL", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
addPolicyPremiumBillAllocation
Adds a policy premium bill allocation to the policy.
Availability
This method is available for Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Schedule Definition Code. Optional |
In |
String |
Add-on Code. Optional |
In |
String |
Enrollment Product Code. Optional |
In |
String |
Insurable Class Code. Optional |
In |
String |
Enrollment Product Category Code. Optional |
In |
String |
Premium Schedule Type Code. Optional |
In |
Date |
The startDate of the new policyPremiumBillAllocation. Mandatory |
In |
Boolean |
Active? Mandatory |
Example
policy.addPolicyPremiumBillAllocation("", "", "GOLD", "", "", "", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time),true)
copy
Creates a copy of the object that this method is called on. It creates a deep copy of the object along with its details. If the object to be copied is policyEnrollmentProduct, then it will also copy the parameterValues defined at the policyEnrollmentProduct level.
Availability
This method is available for PolicyEnrollmentProduct, Policyholder, PolicyContractPeriod, PolicyCollectionSetting, PolicyBrokerAgent, PolicyAddOn and PolicyPremiumBillAllocation. Its purpose is to have the possibility to create a copy of an existing object (of the above mentioned types) and add it to the respective parent. This can be useful for bulk updates functionality where new objects need to be created for new time periods that are usually copies of original rows except for a few details (e.g. dates).
Parameters
In / Out | Type | Description |
---|---|---|
Out |
<Same Object Type that the method is called on> |
This will be same object type that the method is called on. |
Example
def newPolicyEnrollmentProduct = existingPolicyEnrollmentProduct.copy()
copyAndEndDateContractPeriod
Creates a copy of the latest policyContractPeriod. The contract period duration of the new contract period is same as that of the latest policyContractPeriod that is copied. The newly created copy is added to the policyContractPeriod detail list.
If there is no existing contractPeriod detail on the policy, nothing happens and the method returns null.
Availability
This method is available on Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
The startDate of the new policyContractPeriod. Mandatory |
Out |
Policy Contract Period |
The newly created copy of the policyContractPeriod. |
Example
def newPolicyContractPeriod = policy.copyAndEndDateContractPeriod(new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
copyAndEndDateEnrollmentInsurableClass
Creates a copy of the latest policy enrollment insurable class with the passed in insurable class and startDate. It endDates the latest policy enrollment insurable class if the current endDate is null or is on or after the date provided as a parameter to the method. If the current endDate of the most latest policy enrollment insurable class is before the passed in parameter date, then no change happens to the endDate. The newly created copy is added to the policy enrollment insurable class list. If the passed in insurable class does not exist in the system, the dynamic logic using this method fails with a technical error (OHI-DYLO-007).
If there is no existing policy enrollment insurable class on the policy, nothing happens and the method returns null.
Availability
This method is available on Policy Enrollment .
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The insurable class code. Mandatory |
In |
Date |
The startDate of the new policy enrollment insurable class. Mandatory |
Out |
Policy Enrollment Insurable Class |
The newly created copy of the policy enrollment insurable class |
Example
def newPolicyEnrollmentInsurableClass = policyEnrollment.copyAndEndDatePolicyEnrollmentInsurableClass("ADULT", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
copyAndEndDatePolicyholder
Creates a copy of the latest policyholder with the passed in policyholder and startDate. It endDates the most latest policyholder if the current endDate is null or is on or after the date provided as a parameter to the method. If the current endDate of the most latest policyholder is before the passed in parameter date, then no change happens to the endDate. The newly created copy is added to the policyholder detail list. If the passed in policyholder does not exist in the system, the dynamic logic using this method fails with a technical error (OHI-DYLO-007).
If there is no existing policyholder on the policy, nothing happens and the method returns null.
Availability
This method is available on Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The policyholder code (person code). Mandatory |
In |
Date |
The startDate of the new policyholder. Mandatory |
Out |
Policyholder |
The newly created copy of the policyholder |
Example
def newPolicyholder = policy.copyAndEndDatePolicyholder(personCode, new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
copyAndEndDateCollectionSetting
Creates a copy of the latest policyCollectionSetting. It endDates the most latest policy collection setting if the current endDate is null or is on or after the date provided as a parameter to the method. If the current endDate of the most latest policyCollectionSetting is before the passed in parameter date, then no change happens to the endDate. It also puts the startDate of the new copy as the passed in parameter date. The newly created copy is added to the policyCollectionSetting detail list.
If there is no existing collectionSetting detail on the policy, nothing happens and the method returns null.
Availability
This method is available on Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
The startDate of the new policyCollectionSetting. Mandatory |
Out |
Policy Collection Setting |
The newly created copy of the policyCollectionSetting. |
Example
def newPolicyCollectionSetting = policy.copyAndEndDateCollectionSetting(new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
copyAndEndDateBrokerAgent
Creates a copy of the latest policyBrokerAgent with the passed in broker, agent and startDate. It endDates the most latest policyBrokerAgent if the current endDate is null or is on or after the date provided in a parameter to the method. If the current endDate of the most latest policyBrokerAgent is before the passed in parameter date, then no change happens to the endDate. The newly created copy is added to the policyBrokerAgent detail list.
If the passed in broker or agent does not exist in the system, the dynamic logic using this method fails with a technical error (OHI-DYLO-007).
If there is no existing brokerAgent detail on the policy, nothing happens and the method returns null.
Availability
This method is available on Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The broker code of the new policyBrokerAgent. |
In |
String |
The agent code of the new policyBrokerAgent. |
In |
Date |
The startDate of the new policyBrokerAgent. Mandatory |
Out |
Policy Broker Agent |
The newly created copy of the policyBrokerAgent |
Example
def newPolicyBrokerAgent = policy.copyAndEndDateBrokerAgent("BROKERA", null, new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
copyAndEndDateEnrollmentProduct
This method creates a copy of the policy enrollment product that refers to the enrollment product with the code as specified in the first parameter, that has a start date before the specified date (third parameter) and an end date on or after the specified date (third parameter). On the newly created policy enrollment product all fields (fixed fields [1], dynamic fields and dynamic records) are copied from the existing policy enrollment product.
The policy add-ons that are time valid on or after the specified date are copied (all fixed [2] and dynamic fields) if the 'copy add-ons' flag (fourth parameter) is set to true. Note that if multiple policy add-ons exist for the same add-on that are time valid on or after the specified date, only the one with the latest start date is copied.
The parameter values that are time valid on the specified date are copied (all fixed* and dynamic fields) if the 'copy parameter values' flag (fifth parameter) is set to true. Note that if multiple parameter values for the same parameter alias exist that are time valid on or after the specified date, only the one with the latest start date is copied. If this flag is set to false, parameter values are created [3] with the default values as configured on the new product (if applicable).
The policy enrollment product that has been copied is end dated one day before the specified date, including:
-
its policy add-ons that have a start date before the specified date and an end date on or after the specified date
-
the policy add-ons that start on or after the specified date are removed
-
its parameter values that have a start date before the specified date and an end date on or after the specified date
-
the parameter values that start on or after the specified date are removed. If the enrollment product code of the new policy enrollment product does not exist, the dynamic logic using this method will fail with a technical error (OHI-DYLO-007). If no policy enrollment product can be found based on the specified code and date, nothing happens and the method returns null.
Availability
This method is available on object policy enrollment.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The enrollment product code of the policy enrollment product that needs to be copied and end dated (mandatory) |
In |
String |
The enrollment product code of the new policy enrollment product (mandatory) |
In |
Date |
The start date of the new policy enrollment product (mandatory) |
In |
Boolean |
The flag to copy policy add-ons (mandatory) |
In |
Boolean |
The flag to copy parameter values (mandatory) |
Out |
Policy Enrollment Product |
The newly created policy enrollment product |
Example
def newPolicyEnrollmentProduct = policyEnrollment.copyAndEndDateEnrollmentProduct("EPA1", "EPA2", new java.sql.Date(Date.parse("yyyy-MM-dd", "2018-01-01").time), false, false)
copyAndEndDatePolicyGroupAccount
Creates a copy of the latest policyGroupAccount with the passed in policyGroupAccount and startDate. It endDates the latest policyGroupAccount if the current endDate is null or is on or after the date provided in a parameter to the method. If the current endDate of the latest policyGroupAccount is before the passed in parameter date, then no change happens to the endDate. The newly created copy is added to the policyGroupAccount detail list.
If the passed in groupAccount not exists in the system, the dynamic logic using this method fails with a technical error(OHI-DYLO-007).
If there is no existing policyGroupAccount detail on the policy, nothing happens and the method returns null.
Availability
This method is available on Policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The group account code of the new policyGroupAccount. Mandatory |
In |
Date |
The startDate of the new policyGroupAccount. Mandatory |
Out |
Policy Group Account |
The newly created copy of the policyGroupAccount |
Example
def newPolicyGroupAccount = policy.copyAndEndDatePolicyGroupAccount("ORCL", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
getAge
Calculates the age of a person at a given date. Returns the age in years.
Availability
This method is only available for person objects.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
As of date for time validity (optional in case the signature defines a default date) |
Out |
Integer |
Age of the invoking person on the as-of date |
Example
def age = person.getAge()
getCalculationResult
This method returns the newest calculation result for the most recent calculation period belonging to the policy (across policy versions). This is done by filtering the calculation results:
-
Find all calculation results that have the same GID as the policy, then from those calculation results:
Availability
This method is available for object policy.
Parameters
In / Out | Type | Description |
---|---|---|
Out |
CalculationResult |
The calculation result |
getCalendarYearDays
This method returns the number of days of a calendar year based on a reference date:
-
If the reference data falls within a policy contract period, the number of days that makes up the year is set based on the inclusion of the the 29th of February: if the contract period includes the 29th of February the number of days is set to 366, else it is set to 365
-
If the reference date does not fall within a policy contract period, the number of days that makes up the year is the number of days of the calendar year of the reference date Availability
This method is available for object policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
Reference date (mandatory) |
Out |
Number |
The number of days of the calendar year |
Example
This method can for example be used to calculate the daily premium withinAdjustment dynamic logic functions.
def dailyPremium = yearlyAmount / policyEnrollmentProduct.policyEnrollment.policy.getCalendarYearDays(referenceDate)
getDerivedEnrollmentProduct
This method returns the enrollment product that is linked to the policy enrollment product - directly or indirectly (through the group account product in case of policy with a group account)
Availability
This method is available for object policyEnrollmentProduct
Parameters
In / Out | Type | Description |
---|---|---|
Out |
EnrollmentProduct |
The enrollment product |
getGroupAccount
This method returns the group account the policy belongs to on a given date. If no date is given the method returns the group account the policy belongs to on system date. If the policy does not belong to a group account on the given date (or system date) the method returns null.
Availability
This method is available for object policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
As of date for time validity. Optional. |
Out |
Group Account |
The group account |
Example
def polGroupAccount = policy.getGroupAccount(new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time))
getPolicyAccountBalance
This method returns the sum of all non-reversed transactions that meet the criteria. The method returns a money object, the currency of this money object is the currency listed on the policy account definition.
The AsOfDate specifies, in combination with the policy account definition’s period, the period for which the account balance is calculated. If the asOfDate is omitted, the account balance for the system date is returned.
The method returns a balance 0 if one or more of the following is true:
-
the actual sum of the policy account transactions is 0
-
no policy account transactions are found that meet the criteria
-
no policy account is found that meets the criteria
-
the policy account definition, policy account transaction type or person in the criteria are unknown. If the policy account definition in the criteria is unknown the returned balance is 0. The currency of that balance is set to the system’s default currency.
This method does not differentiate between the situation where the actual sum of the found transactions is 0, and the situation where no policy account could be found. To check for the existence of a policy account use the predefined method getPolicyAccountNumber.
Availability
This method is available for object policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Policy Account Definition Code. Mandatory |
In |
String |
Policy Account Transaction Type Code. Optional |
In |
Date |
asOfDate. Optional |
In |
String |
Policy Enrollment Person Code. Optional |
Out |
Money |
The balance of the account |
Example
def newPolicyAccountBalance = policy.getPolicyAccountBalance("HSA", "PREM", new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time),"Person123")
getPolicyAccountNumber
This method returns the account number of the policy account that meets the criteria.
Availability
This method is available for object policy.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Policy Account Definition Code. Mandatory |
In |
String |
Policy Enrollment Person Code. Optional |
Out |
String |
Policy Account Number |
Example
def newPolicyAccountNumber = policy.getPolicyAccountNumber("HSA","Person123")
If the person code is omitted, only a policy account of the definition level Policy will be returned (if it exists). If the person code is filled, only a policy account of the definition level Person will be returned (if it exists). If no policy account matches the criteria the method returns null.
getPolicyEnrollmentProducts
This method searches for the relevant policy enrollment products for a person or object within the system. It returns the list of policy enrollment products that have an overlap in time validity with the time period as given by the start date and end date. These policy enrollment products belong to the latest approved version of the policies.
Availability
This method is available for insurable entities
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
Start date for time validity. Optional. |
In |
Date |
End date for time validity. Optional. |
Out |
List of PolicyEnrollmentProducts |
The policy enrollment product list |
Example
peps = insurableEntity.getPolicyEnrollmentProducts(new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-01-01").time) ,new java.sql.Date(Date.parse("yyyy-MM-dd", "2016-12-31").time)) peps.each{ pep -> if (pep.policyEnrollment.policy.lineOfBusiness.code != "HEALTH') { return } ...
In the example the getPolicyEnrollmentProducts method fills a list with enrollment products for all policies that this insurable entity is enrolled on, where the enrollment products have a time validity that overlaps with the period 1 Jan 2016 to 31 December 2016.
In the next step for each of the entries in the list it is checked if the policy is for the line of business 'HEALTH'.
getPolicies
This method searches for the latest end-state (approved or canceled) version of the policies for a person or object within the system. It returns the list of policies where the person or object has a policy enrollment.
Availability
This method is available for object insurable entity
Parameters
In / Out | Type | Description |
---|---|---|
Out |
List of Policies |
The policy list |
Example
pols = insurableEntity.getPolicies pols.each{ pol -> if (pol.lineOfBusiness.code != "HEALTH') { return } ...
In the example the getPolicies method fills a list with policies that this insurable entity is enrolled on. In the next step for each of the entries in the list it is checked if the policy is for the line of business 'HEALTH'.
getPolicyBillingAccounts
This method returns the policy billing accounts of a policy.
Availability
This method is available for policy
Parameters
In / Out | Type | Description |
---|---|---|
Out |
List<PolicyBillingAccount> |
The policy billing account list |
Example
List<PolicyBillingAccount> policyBillingAccounts = policy.getPolicyBillingAccounts()
getPolicyCalculationPeriods
This method searches for the relevant policy calculation periods. It returns the policy calculation periods that have an overlap with the time period as given by the start date and end date.
Due to the possible large number of results, the method does not return a list containing all policy calculation periods, but only a subset (called a page). The number of entries per page is controlled by property ohi.dynamiclogic.pagesize.
Availability
This method is available for policy
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
Start date for time validity. |
In |
Date |
End date for time validity. Optional. |
In |
Integer |
Number of the page to be retrieved. Value >= 0 |
Out |
List<PolicyCalculationPeriod> |
The subset of the policy calculation periods that belong to page N. If the page size is 50, and the page is 3, the periods returned are 151-200 (assuming they exist) |
Example
int page = 0 while (true) { def periods = policy.getPolicyCalculationPeriods(exampleStartDate, exampleEndDate, page++) if (periods.size() == 0) { break } periods.each { policyCalculationPeriod -> // Execute any further logic, e.g. set a (dynamic) field policyCalculationPeriod.exampleField = exampleValue } }
Every invocation of getPolicyCalculationPeriods does a separate database query. When the policy calculation periods are being mutated by some other process simultaneously, that impacts the results of next pages retrieved. |
getProviderGroups
This method searches for the assigned provider groups of a policy enrollment product. It returns the list of enrollment product provider groups or group account product provider groups that have an overlap in time validity with the time period as given by the start date and end date. If for a policy enrollment product both enrollment product provider groups and group account provider groups are found that have an overlap in time validity with the time period as given by the start date and end date only the group account product provider groups are returned.
Availability
This method is available for policy enrollment products.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
Start date for time validity. Optional. |
In |
Date |
End date for time validity. Optional. |
Out |
List of EnrollmentProductProviderGroups or GroupAccountProductProviderGroups |
The assigned provider group list |
Refer to the Enrollment Response Integration Point specification in the integration guide for examples.
inProviderGroup
Checks whether a provider is part of a provider group at a certain point in time. Returns true when that is the case, false otherwise.
An organization provider is part of a provider group if:
-
the organization provider is affiliated to the provider group OR
-
the organization provider is part of an organization provider that is affiliated to the provider group An individual provider is part of a provider group if:
-
the individual provider is affiliated to the provider group The affiliations must be valid at the as of date in all cases.
Availability
This method is available for provider objects, both individual and organizational providers.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Code of the provider group. Mandatory. |
In |
Date |
As of date for time validity (optional in case the signature defines a default date) |
Out |
Boolean |
True if the invoking provider belongs to the group on the as-of date |
Example
individualProvider.inProviderGroup('CORE')
getCountryRegions (provider)
Get the country regions of a provider. Use addresses at given point in time. Returns the country regions if found, null otherwise.
Availability
This method is available on provider objects.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
As of date for time validity (optional in case the signature defines a default date) |
Out |
CountryRegionList |
For individual providers: returns the country regions of the invoking provider’s rendering addresses (specified on the related service addresses) on the as-of date. For organization providers: returns the country regions of the invoking provider’s service addresses on the as-of date. |
Example
countryRegions = provider.getCountryRegions()
getTitles
This method returns a string containing all the titles of the person or individual provider separated by white spaces. The titles are sorted on display order. If no display order is available or several titles have the same display order, the alphabetic ordering of the title applies.
Availability
This method is available on person and individual provider objects.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
'A' returns all post-name titles, 'B' returns all pre-name titles. |
Out |
String |
Space separated list of the invoking person’s titles |
Example
return person.getTitles('B') + ' ' + person.name + ' ' + person.getTitles('A')
hasSpecialty
Checks whether a provider has a specialty at a certain point in time. Returns true when that is the case, false otherwise.
Availability
This method is available for provider objects, both individual and organizational providers.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
Code of the specialty |
In |
Date |
As of date for time validity (optional in case the signature defines a default date) |
Out |
Boolean |
True the invoking provider has a provider specialty that matches the code on the as-of date |
Example
provider.hasSpecialty('DERMATOLOGY')
setField
The usual way to assign a value is to use the assignment operator, for example:
groupAccount.displayName = 'A group account description'
In the case of a dynamic field that is in a FlexCode Set, a direct assignment is not possible because you need to specify the code of the FlexCode Definition.
This method sets the value for a dynamic field that is in a FlexCode Set. The field has to be single value and non-time-valid.
Availability
This method is only available for functions that allow a value to be set within the dynamic logic.
Parameters
In / Out | Type | Description |
---|---|---|
In |
String |
The name of the field which is to be set. |
In |
String, Date or Number |
The value of the field to be set. For flex codes, the key field value. |
In |
String |
Code of the FlexCode Definition |
Example
This example assigns a value to the groupSize field on the groupAccount. The field groupSize is of type groupSizeCatagory which is defined as flex code set 'GROUP_SIZE_CATAGORY. Typical values for the group size are 'SMALL', 'MEDIUM', and 'LARGE'. We can use the following code to set the MEDIUM groupsize for the group account in context.
groupAccount.setField('groupSize','MEDIUM','GROUP_SIZE_CATAGORY')
setPersonCoveredService
This method replaces the list of person covered services for a person using enrollment information of that person and product covered services.
The method takes the following input parameters:
-
Reference Input Date (optional)
-
Number of Portability days (optional)
-
Product Code for Transfer Certificates (optional)
Step 1
The method removes all person covered services for the person in context that have a time overlap with, or start date after the reference input date except for those that have Locked? set to Yes and except for the transfer certificates (having the transfer certificates product code).
Typically, the reference input date would be set to restrict the replacement of the person covered services as of a specific date. If not set, the method removes all person covered service for this person.
Step 2
-
The method collects the enrollment products for which the person is or was enrolled on or after the reference input date.
-
The method then collects the product(s) each of the enrollment products refer to (through enrollment product detail), copying the time validity of the enrollment product
-
If one enrollment product links to multiple products, the method selects all linked products adding the time validity of the enrollment product for all linked products
-
If multiple enrollment products link to the same product, the method uses the total time validity of the enrollment products for the product. This could be one continuous time window or multiple time windows.
The result of this step so far is a list of products (not enrollment products!) the member was enrolled on with a start- and end date. From here we will call them product time windows.
-
-
For each product time window the method adds a copy of all the Product Covered Services for the referred product valid on the current date to the Person Covered Services.
-
Copy the Start and End Date from the 'Product Time Window' selected in the previous step
-
Copy the product code, service option service code, covered service type, covered service tier, and score from the product covered service
-
Set the Indicator Locked to No
-
Leave Indicator Waived and Waiver Reason empty
-
Set the wait start date according the Determine Wait Start Date description below.
-
Determine Wait Start Date
Default
The method checks if a previous connecting product time window exists.
'Previous connecting' means:
-
The start date of the current product time window is the end date + 1 of the previous product.
-
The product in the previous product time window has a product covered service with the same service option service code but an equal or better score as the person covered service the method is currently creating.
In order to find the earliest start date of the service in a previous product, the system keeps moving back in the product history, as long as the previous covered service has an equal or better score.
If the previous covered service has a lower score than the current covered service, the system does not move back and considers the start date of the current product time window as the waiting start date.
Please see Examples for a more detailed examples on how the wait start date is determined.
In case of Portabillity (Transfer Certificates)
If the 'Number of Portability Days' and the 'Product Code for Transfer Certificates' parameters of the predefined method are specified, the method also searches for person covered services for the specified Transfer Certificate product [4] [5].
If the start date of the current product time window is between start date + 1 and end date + 1 + Number of Portability Days, of the transaction certificate person covered service, the method uses the start date of that person covered service as wait start date for the new person covered service.
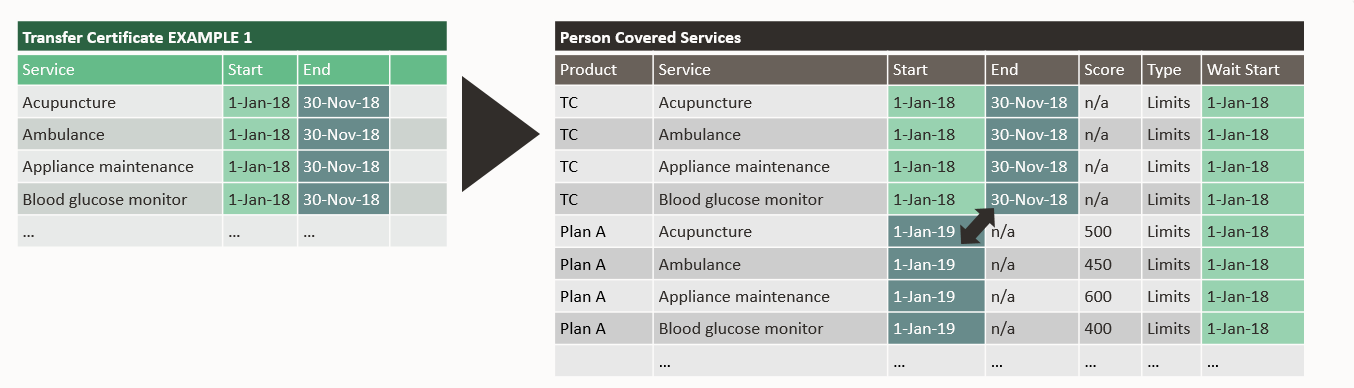
If the start date is not within that range, the system uses the start date of the current product time window.
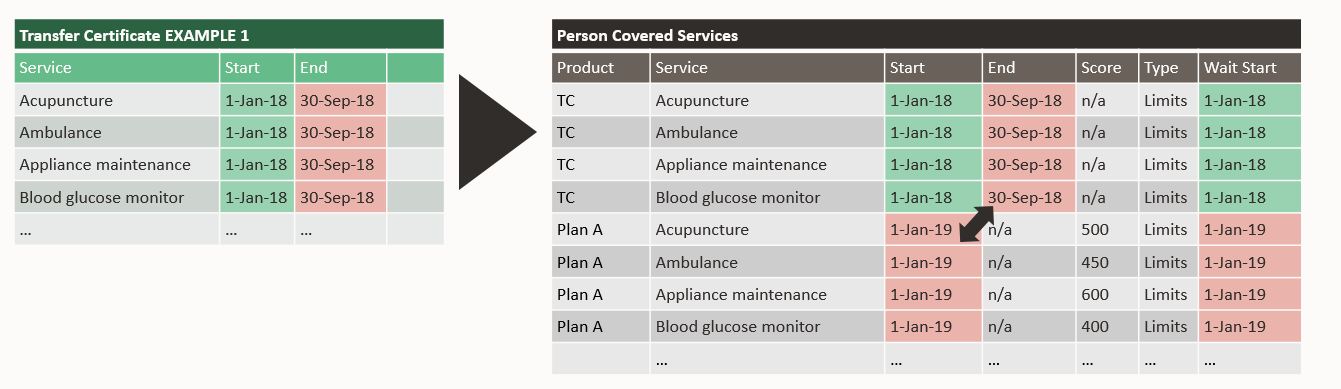
With Locked Person Covered Services in Place.
If a to-be-created Person Covered Service overlaps with an existing locked Person Covered Service, the method will adjust the start- or enddate of the new Person Covered Service in such way that the date of the new and existing person Covered Service(s) align with the locked one.
The method will not automatically lock the new person covered service.
With Locked and Waived Person Covered Services in Place.
If a to-be-created Person Covered Service overlaps with an existing locked Person Covered Service that is Waived, the additionally created person covered service(s) (aligning with the existing one), are also waived with the same waive reason and they are locked.
Availability
This method is only available in the context of a dynamic logic function of signature 'person covered service'.
Parameters
In / Out | Type | Description |
---|---|---|
In |
Date |
The date as of which the person covered services need to be set |
In |
String |
The max number of gap days between products |
In |
String |
The code of the product that is used for transfer Certificates |
Example
person.setPersonCoveredService(new java.sql.Date(Date.parse("yyyy-MM-dd", "2019-01-01").time),60,'TC')
split
This method splits a policy calculation period into multiple smaller ones on the provided input dates. An input date represent the start of a new policy calculation period, so the preceding policy calculation period is ended one day before.
For example, splitting the policy calculation period of January 2010 on the 10th, will result in two policy calculation periods:
-
From the 1st to the 9th
-
From the 10th to the 31th
If the same policy calculation period of January 2010 is split on the both the 10th and the 20th of January, the result is three policy calculation periods:
-
From the 1st to the 9th
-
From the 10th to the 19th
-
From the 20th to the 31th
If the none of the input dates lay within the policy calculation period, the returned list simply contains the original policy calculation period.
The "Days" attribute is adjusted on the resulting policy calculation periods by linear interpolation. All other fields, including dynamic fields, are copied from the original policy calculation period to the new ones.
Availability
This method is available for policy calculation periods.
Parameters
In / Out | Type | Description |
---|---|---|
In |
A single Date, multiple Dates, or a List<Date> |
The date(s) on which the policy calculation period should be split |
Out |
List<PolicyCalculationPeriod> |
The split policy calculation periods |
Example 1: single date
import java.sql.Date Date myDate = Date.valueOf("2000-01-01") List<PolicyCalculationPeriod> splitPolicyCalculationPeriods = policyCalculationPeriod.split(myDate) assert splitPolicyCalculationPeriods.size() == 2 assert splitPolicyCalculationPeriods[0].startDate == policyCalculationPeriod.startDate assert splitPolicyCalculationPeriods[0].endDate == myDate - 1 assert splitPolicyCalculationPeriods[1].startDate == myDate assert splitPolicyCalculationPeriods[1].endDate == policyCalculationPeriod.endDate
Example 2: multiple dates
import java.sql.Date Date myDate1 = Date.valueOf("2000-01-01") Date myDate2 = Date.valueOf("2000-01-02") Date myDate3 = Date.valueOf("2000-01-03") List<PolicyCalculationPeriod> splitPolicyCalculationPeriods = policyCalculationPeriod.split(myDate1, myDate2, myDate3) assert splitPolicyCalculationPeriods.size() == 4
Example 3: list
import txt.sql.Date Date myDate1 = Date.valueOf("2000-01-01") Date myDate2 = Date.valueOf("2000-01-02") List<Date> myDateList = [myDate1, myDate2] List<PolicyCalculationPeriod> splitPolicyCalculationPeriods = policyCalculationPeriod.split(myDateList) assert splitPolicyCalculationPeriods.size() == myDateList.size() + 1