Parametric search is the ability to restrict the search based on properties of the item type being searched for. For example, you could enable users to search for products made by a certain manufacturer. The search results would return only those product repository items whose manufacturer
property is set to the specified value.
The following figure illustrates a search form that allows the user to restrict the search to products from a single manufacturer:
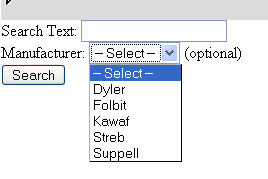
The following JSP example creates a page that includes the form controls shown above.
<%@ taglib prefix="c" uri="http://java.sun.com/jstl/core" %> <%@ taglib prefix="dspel" uri="http://www.atg.com/taglibs/daf/dspjspELTaglib1_0" %> <dspel:page> <script language="javascript"> /** * Changes the page number. **/ function changePage(page) { var form = document.forms['searchForm']; if(form == null) return false; var input = form.elements['pageNum']; if(input == null) return false; input.value=page; return true; } </script> <c:set var="formHandlerPath" value= "/atg/commerce/search/ProductCatalogQueryFormHandler"/> <dspel:getvalueof var="thisPage" bean="/OriginatingRequest.requestURI"/> <dspel:form formid="searchForm" name="searchForm" method="POST" action="${thisPage}"> <!-- The page number --> <dspel:getvalueof var="pageNum" bean="${formHandlerPath}.requestAttributes.pageNum"/> <c:if test="${empty pageNum}"><c:set var="pageNum" value="0" scope="request"/></c:if> <dspel:input type="hidden" name="pageNum" bean="${formHandlerPath}.requestAttributes.pageNum" value="${pageNum}"/> <!-- The text input --> Search Text: <dspel:input type="text" name="question" bean="${formHandlerPath}.question" beanvalue="${formHandlerPath}.question" onchange="changePage(0);"/> <!-- The parametric search control --> <!-- This generates the following XML query constraint --> <!-- <prop type="string" name="manufacturer.$repositoryId" op="equals" case="false">Kawaf</prop> --> <dspel:input type="hidden" bean="${formHandlerPath}.documentSetsBuilder.tags.str1.tagname" value="prop" /> <dspel:input type="hidden" bean="${formHandlerPath}.documentSetsBuilder.tags.str1.opts.generate" value="ifvalue:body" /> <dspel:input type="hidden" bean="${formHandlerPath}.documentSetsBuilder.tags.str1.attr.type" value="string"/> <dspel:input type="hidden" bean="${formHandlerPath}.documentSetsBuilder.tags.str1.attr.name" value="manufacturer.$repositoryId"/> <dspel:input type="hidden" bean="${formHandlerPath}.documentSetsBuilder.tags.str1.attr.op" value="equal"/> <dspel:input type="hidden" bean="${formHandlerPath}.documentSetsBuilder.tags.str1.attr.case" value="false"/> Manufacturer: <dspel:select multiple="false" bean="${formHandlerPath}.documentSetsBuilder.tags.str1.body" onclick='changePage(0);'> <dspel:importbean var="CatalogTools" bean="/atg/commerce/catalog/CatalogTools"/> <dspel:droplet var="possibleValues" name= "/atg/dynamo/droplet/PossibleValues"> <dspel:param name="itemDescriptorName" value="manufacturer"/> <dspel:param name="repository" value="${CatalogTools.catalog}"/> <dspel:param name="sortProperties" value="+displayName"/> <dspel:oparam name="output"> <dspel:option value="">-- Select --</dspel:option> <c:forEach var="manufacturer" items="${possibleValues.values}"> <dspel:oparam name="output"> <dspel:tomap var="manufacturerMap" value="${manufacturer}"/> <dspel:option value="${manufacturer.repositoryId}"> <c:out value="${manufacturerMap.displayName}"/> </dspel:option> </dspel:oparam> </c:forEach> </dspel:oparam> </dspel:droplet> </dspel:select> (optional) <!-- submit button --> <dspel:input type="submit" bean="${formHandlerPath}.search" name="submit" value="Search" priority="-20"/> </dspel:form> </dspel:page>