11 Scroll Pane
In this chapter, you learn how to build scroll panes in your JavaFX applications.
Scroll panes provide a scrollable view of UI elements. This control enables the user to scroll the content by panning the viewport or by using scroll bars. A scroll pane with the default settings and the added image is shown in Figure 11-1.
Creating a Scroll Pane
Example 11-1 shows how to create this scroll pane in your application.
Example 11-1 Using a Scroll Pane to View an Image
Image roses = new Image(getClass().getResourceAsStream("roses.jpg")); ScrollPane sp = new ScrollPane(); sp.setContent(new ImageView(roses));
The setContent
method defines the node that is used as the content of this scroll pane. You can specify only one node. To create a scroll view with more than one component, use layout containers or the Group
class. You can also specify the true
value for the setPannable
method to preview the image by clicking it and moving the mouse cursor. The position of the scroll bars changes accordingly.
Setting the Scroll Bar Policy for a Scroll Pane
The ScrollPane
class provides a policy to determine when to display scroll bars: always, never, or only when they are needed. Use the setHbarPolicy
and setVbarPolicy
methods to specify the scroll bar policy for the horizontal and vertical scroll bars respectively. Thus, in Example 11-2 the vertical scroll bar will appear, but not the horizontal scroll bar.
Example 11-2 Setting the Horizontal and Vertical Scroll Bar Policies
sp.setHbarPolicy(ScrollBarPolicy.NEVER); sp.setVbarPolicy(ScrollBarPolicy.ALWAYS);
As a result, you can only scroll the image vertically, as shown in Figure 11-2.
Figure 11-2 Disabling the Horizontal Scroll Bar
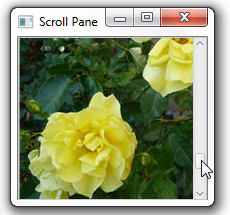
Description of "Figure 11-2 Disabling the Horizontal Scroll Bar "
Resizing Components in the Scroll Pane
When designing a UI interface, you might need to resize the components, so that they match the width or height of the scroll pane. Set either the setFitToWidth
or setFitToHeight
method to true
to match a particular dimension.
The scroll pane shown in Figure 11-3 contains radio buttons, a text box, and a password box. The size of the content exceeds the predefined size of the scroll pane and a vertical scroll bar appears. However, because the setFitToWidth
method sets true
for the scroll pane, the content shrinks in width and never scrolls horizontally.
Figure 11-3 Fitting the Width of the Scroll Pane
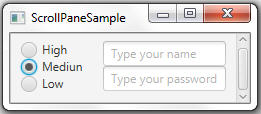
Description of "Figure 11-3 Fitting the Width of the Scroll Pane"
By default, both FIT_TO_WIDTH
and FIT_TO_HEIGHT
properties are false,
and the resizable content keeps its original size. If you remove the setFitToWidth
methods from the code of this application, you will see the output shown in Figure 11-4.
Figure 11-4 Default Properties for Fitting the Content
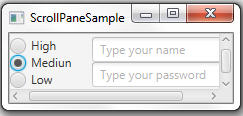
Description of "Figure 11-4 Default Properties for Fitting the Content"
The ScrollPane
class enables you to retrieve and set the current, minimum, and maximum values of the contents in the horizontal and vertical directions. Learn how to use them in your applications.
Sample Application with a Scroll Pane
Example 11-3 uses a scroll pane to display a vertical box with images. The VVALUE
property of the ScrollPane
class helps to identify the currently displayed image and to render the name of the image file.
Example 11-3 Using a Scroll Pane to View Images
import javafx.application.Application; import javafx.beans.value.ObservableValue; import javafx.scene.Scene; import javafx.scene.control.Label; import javafx.scene.control.ScrollPane; import javafx.scene.image.Image; import javafx.scene.image.ImageView; import javafx.scene.layout.Priority; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class Main extends Application { final ScrollPane sp = new ScrollPane(); final Image[] images = new Image[5]; final ImageView[] pics = new ImageView[5]; final VBox vb = new VBox(); final Label fileName = new Label(); final String [] imageNames = new String [] {"fw1.jpg", "fw2.jpg", "fw3.jpg", "fw4.jpg", "fw5.jpg"}; @Override public void start(Stage stage) { VBox box = new VBox(); Scene scene = new Scene(box, 180, 180); stage.setScene(scene); stage.setTitle("Scroll Pane"); box.getChildren().addAll(sp, fileName); VBox.setVgrow(sp, Priority.ALWAYS); fileName.setLayoutX(30); fileName.setLayoutY(160); for (int i = 0; i < 5; i++) { images[i] = new Image(getClass().getResourceAsStream(imageNames[i])); pics[i] = new ImageView(images[i]); pics[i].setFitWidth(100); pics[i].setPreserveRatio(true); vb.getChildren().add(pics[i]); } sp.setVmax(440); sp.setPrefSize(115, 150); sp.setContent(vb); sp.vvalueProperty().addListener((ObservableValue<? extends Number> ov, Number old_val, Number new_val) -> { fileName.setText(imageNames[(new_val.intValue() - 1)/100]); }); stage.show(); } public static void main(String[] args) { launch(args); } }
Compiling and running this application produces the window shown in Figure 11-5.
The maximum value of the vertical scroll bar is equal to the height of the vertical box. The code fragment shown in Example 11-4 renders the name of the currently displayed image file.
Example 11-4 Tracking the Change of the Scroll Pane's Vertical Value
sp.vvalueProperty().addListener((ObservableValue<? extends Number> ov, Number old_val, Number new_val) -> { fileName.setText(imageNames[(new_val.intValue() - 1)/100]); });
The ImageView
object limits the image height to 100 pixels. Therefore, when new_val.intValue() - 1
is divided by 100, the result gives the index of the current image in the imageNames
array.
In your application, you can also vary minimum and maximum values for vertical and horizontal scroll bars, thus dynamically updating your user interface.
Related API Documentation