8 Text Field
This chapter discusses the capabilities of the text field control.
The TextField
class implements a UI control that accepts and displays text input. It provides capabilities to receive text input from a user. Along with another text input control, PasswordField
, this class extends the TextInput
class, a super class for all the text controls available through the JavaFX API.
Figure 8-1 shows a typical text field with a label.
Creating a Text Field
In Example 8-1, a text field is used in combination with a label to indicate the type of content that should be typed in the field.
Example 8-1 Creating a Text Field
Label label1 = new Label("Name:"); TextField textField = new TextField (); HBox hb = new HBox(); hb.getChildren().addAll(label1, textField); hb.setSpacing(10);
You can create an empty text field as shown in Example 8-1 or a text field with a particular text data in it. To create a text field with the predefined text, use the following constructor of the TextField
class: TextField("Hello World!")
. You can obtain the value of a text field at any time by calling the getText
method.
You can apply the setPrefColumnCount
method of the TextInput
class to set the size of the text field, defined as the maximum number of characters it can display at one time.
Building the UI with Text Fields
Typically, the TextField
objects are used in forms to create several text fields. The application in Figure 8-2 displays three text fields and processes the data that a user enters in them.
The code fragment in Example 8-2 creates the three text fields and two buttons, and adds them to the application's scene by using the GridPane
container. This container is particularly handy when you need to implement a flexible layout for your UI controls.
Example 8-2 Adding Text Fields to the Application
//Creating a GridPane container GridPane grid = new GridPane(); grid.setPadding(new Insets(10, 10, 10, 10)); grid.setVgap(5); grid.setHgap(5); //Defining the Name text field final TextField name = new TextField(); name.setPromptText("Enter your first name."); GridPane.setConstraints(name, 0, 0); grid.getChildren().add(name); //Defining the Last Name text field final TextField lastName = new TextField(); lastName.setPromptText("Enter your last name."); GridPane.setConstraints(lastName, 0, 1); grid.getChildren().add(lastName); //Defining the Comment text field final TextField comment = new TextField(); comment.setPromptText("Enter your comment."); GridPane.setConstraints(comment, 0, 2); grid.getChildren().add(comment); //Defining the Submit button Button submit = new Button("Submit"); GridPane.setConstraints(submit, 1, 0); grid.getChildren().add(submit); //Defining the Clear button Button clear = new Button("Clear"); GridPane.setConstraints(clear, 1, 1); grid.getChildren().add(clear);
Take a moment to study the code fragment. The name
, lastName
, and comment
text fields are created by using empty constructors of the TextField
class. Unlike Example 8-1, labels do not accompany the text fields in this code fragment. Instead, prompt captions notify users what type of data to enter in the text fields. The setPromptText
method defines the string that appears in the text field when the application is started. When Example 8-2 is added to the application, it produces the output shown in Figure 8-3.
Figure 8-3 Three Text Fields with the Prompt Messages
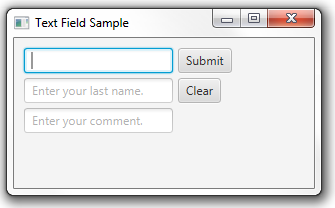
Description of "Figure 8-3 Three Text Fields with the Prompt Messages"
The difference between the prompt text and the text entered in the text field is that the prompt text cannot be obtained through the getText
method.
In real-life applications, data entered into the text fields is processed according to an application's logic as required by a specific business task. The next section explains how to use text fields to evaluate the entered data and generate a response to a user.
Processing Text Field Data
As mentioned earlier, the text data entered by a user into the text fields can be obtained by the getText
method of the TextInput
class.
Study Example 8-3 to learn how to process the data of the TextField
object.
Example 8-3 Defining Actions for the Submit and Clear Buttons
//Adding a Label final Label label = new Label(); GridPane.setConstraints(label, 0, 3); GridPane.setColumnSpan(label, 2); grid.getChildren().add(label); submit.setOnAction((ActionEvent e) -> { if ( (comment.getText() != null && !comment.getText().isEmpty()) ) { label.setText(name.getText() + " " + lastName.getText() + ", " + "thank you for your comment!"); } else { label.setText("You have not left a comment."); } }); clear.setOnAction((ActionEvent e) -> { name.clear(); lastName.clear(); comment.clear(); label.setText(null); });
The Label
control added to the GridPane
container renders an application's response to users. When a user clicks the Submit button, the setOnAction
method checks the comment
text field. If it contains a nonempty string, a thank-you message is rendered. Otherwise, the application notifies a user that the comment message has not been left yet, as shown in Figure 8-4.
Figure 8-4 The Comment Text Field Left Blank
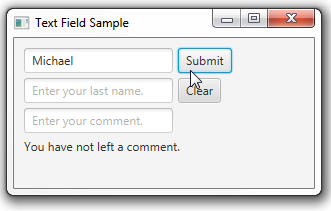
Description of "Figure 8-4 The Comment Text Field Left Blank"
When a user clicks the Clear button, the content is erased in all three text fields.
Review some helpful methods that you can use with text fields.
-
copy()
– transfers the currently selected range in the text to the clipboard, leaving the current selection. -
cut()
– transfers the currently selected range in the text to the clipboard, removing the current selection. -
selectAll()
- selects all text in the text input. -
paste()
– transfers the contents in the clipboard into this text, replacing the current selection.
Related API Documentation