40 Applying Effects to Text
In this chapter you learn how to apply single effects and a chain of effects to text nodes. It includes demo samples to illustrate the APIs being used.
The JavaFX SDK provides a wide set of effects that reside in the javafx.scene.effect
package. For a complete set of available effects, see the API documentation. You can see some of the effects in action in the TextEffects
demo application. This application displays text nodes with various effects. Download the TextEffectsSample.zip
file, extract the files, save them on your computer, and open a NetBeans project in the NetBeans IDE.
Perspective Effect
The PerspectiveTansform
class enables you to imitate a three-dimensional effect for your two-dimensional content. The perspective transformation can map an arbitrary quadrilateral into another quadrilateral. An input for this transformation is your node. To define the perspective transformation, you specify the x and y coordinates of output locations of all four corners. In the TextEffects
application, the PerspectiveTransform effect is set for a group consisting of a rectangle and text as shown in Example 40-1.
Example 40-1
PerspectiveTransform pt = new PerspectiveTransform();
pt.setUlx(10.0f);
pt.setUly(10.0f);
pt.setUrx(310.0f);
pt.setUry(40.0f);
pt.setLrx(310.0f);
pt.setLry(60.0f);
pt.setLlx(10.0f);
pt.setLly(90.0f);
g.setEffect(pt);
g.setCache(true);
Rectangle r = new Rectangle();
r.setX(10.0f);
r.setY(10.0f);
r.setWidth(280.0f);
r.setHeight(80.0f);
r.setFill(Color.BLUE);
Text t = new Text();
t.setX(20.0f);
t.setY(65.0f);
t.setText("Perspective");
t.setFill(Color.YELLOW);
t.setFont(Font.font(null, FontWeight.BOLD, 36));
g.getChildren().add(r);
g.getChildren().add(t);
return g;
Using the setCache(true)
property enables you to improve performance when rendering the node.
You can see the result of the perspective transformation in Figure 40-1.
Figure 40-1 Text with a Perspective Effect
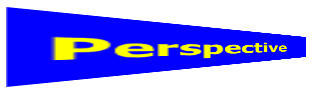
Description of "Figure 40-1 Text with a Perspective Effect"
Blur Effect
The GaussianBlur
class provides a blur effect based on a Gaussian convolution kernel.
Example 40-2 shows a text node with an applied Gaussian blur effect implemented in the TextEffects
application.
Example 40-2
Text t2 = new Text(); t2.setX(10.0f); t2.setY(140.0f); t2.setCache(true); t2.setText("Blurry Text"); t2.setFill(Color.RED); t2.setFont(Font.font(null, FontWeight.BOLD, 36)); t2.setEffect(new GaussianBlur()); return t2;
You can see the result of the Gaussian blur effect in Figure 40-2.
Drop Shadow Effect
To implement a drop shadow effect, use the DropShadow
class. You can specify a color and an offset for the shadow of your text. In the TextEffects
application, the drop shadow effect is applied to the red text and provides a three-pixel gray shadow. You can see the code in Example 40-3.
Example 40-3
DropShadow ds = new DropShadow(); ds.setOffsetY(3.0f); ds.setColor(Color.color(0.4f, 0.4f, 0.4f)); Text t = new Text(); t.setEffect(ds); t.setCache(true); t.setX(10.0f); t.setY(270.0f); t.setFill(Color.RED); t.setText("JavaFX drop shadow..."); t.setFont(Font.font(null, FontWeight.BOLD, 32));
You can see the result of the applied effect in Figure 40-3.
Figure 40-3 Text with a Drop Shadow Effect

Description of "Figure 40-3 Text with a Drop Shadow Effect"
Inner Shadow Effect
An inner shadow effect renders a shadow inside the edges of your content. For text content, you can specify a color and an offset. See how the inner shadow effect with four-pixel offsets in the x and y directions is applied to a text node in Example 40-4.
Example 40-4
InnerShadow is = new InnerShadow(); is.setOffsetX(4.0f); is.setOffsetY(4.0f); Text t = new Text(); t.setEffect(is); t.setX(20); t.setY(100); t.setText("InnerShadow"); t.setFill(Color.YELLOW); t.setFont(Font.font(null, FontWeight.BOLD, 80)); t.setTranslateX(300); t.setTranslateY(300); return t;
The result of the applied effect is shown in Figure 40-4.
Figure 40-4 Text with an Inner Shadow Effect

Description of "Figure 40-4 Text with an Inner Shadow Effect"
Reflection
The Reflection
class enables you to display the reflected version of your text below the original text. You can adjust the view of your reflected text by providing additional parameters such as a bottom opacity value, a fraction of the input to be visible in the reflection, an offset between the input text and its reflection, and a top opacity value. For details, see the API documentation.
The reflection effect is implemented in the TextEffects
application as shown in Example 40-5.
Example 40-5
Text t = new Text(); t.setX(10.0f); t.setY(50.0f); t.setCache(true); t.setText("Reflections on JavaFX..."); t.setFill(Color.RED); t.setFont(Font.font(null, FontWeight.BOLD, 30)); Reflection r = new Reflection(); r.setFraction(0.7f); t.setEffect(r); t.setTranslateY(400); return t;
You can see the text node with the reflection effect in Figure 40-5.
Figure 40-5 Text with a Reflection Effect
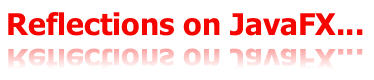
Description of "Figure 40-5 Text with a Reflection Effect"
Combining Several Effects
In the previous section you learned how to apply a single effect to a text node. To further enrich your text content, you can compose several effects and apply a chain of effects to achieve a specific visual result. Consider the NeonSign
application shown in Figure 40-6.
Figure 40-6 The Neon Sign Application Window
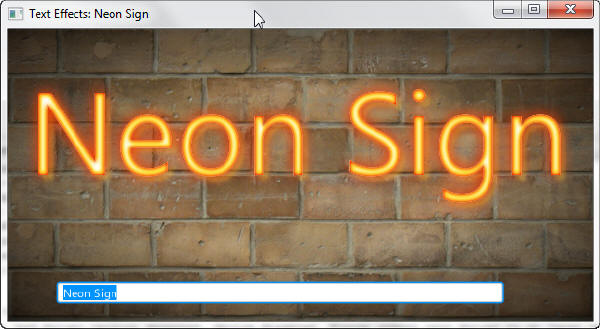
Description of "Figure 40-6 The Neon Sign Application Window"
The graphical scene of the NeonSign
application consists of the following elements:
-
An image of a brick wall used as a background
-
A rectangle that provides a radial gradient fill
-
A text node with a chain of effects
-
A text field used for entering text data
The background is set in an external .css file.
This application uses a binding mechanism to set the text content on the text node. The text property of the text node is bound to the text property of the text field as shown in Example 40-6.
Example 40-6
Text text = new Text(); TextField textField = new TextField(); textField.setText("Neon Sign"); text.textProperty().bind(textField.textProperty());
You can type in the text field and view the changed contents of the text node.
A chain of effects is applied to the text node. The primary effect is a blend effect that uses the MULTIPLY
mode to mix two inputs together: a drop shadow effect and another blend effect, blend1
. Similarly, the blend1
effect combines a drop shadow effect (ds1
) and a blend effect (blend2
). The blend2
effect combines two inner shadow effects. Using this chain of effects and varying color parameters enables you to apply subtle and sophisticated color patterns to text objects. See the code for the chain of effects in Example 40-7.
Example 40-7
Blend blend = new Blend(); blend.setMode(BlendMode.MULTIPLY); DropShadow ds = new DropShadow(); ds.setColor(Color.rgb(254, 235, 66, 0.3)); ds.setOffsetX(5); ds.setOffsetY(5); ds.setRadius(5); ds.setSpread(0.2); blend.setBottomInput(ds); DropShadow ds1 = new DropShadow(); ds1.setColor(Color.web("#f13a00")); ds1.setRadius(20); ds1.setSpread(0.2); Blend blend2 = new Blend(); blend2.setMode(BlendMode.MULTIPLY); InnerShadow is = new InnerShadow(); is.setColor(Color.web("#feeb42")); is.setRadius(9); is.setChoke(0.8); blend2.setBottomInput(is); InnerShadow is1 = new InnerShadow(); is1.setColor(Color.web("#f13a00")); is1.setRadius(5); is1.setChoke(0.4); blend2.setTopInput(is1); Blend blend1 = new Blend(); blend1.setMode(BlendMode.MULTIPLY); blend1.setBottomInput(ds1); blend1.setTopInput(blend2); blend.setTopInput(blend1); text.setEffect(blend);
In this article, you learned how to add text and apply various effects to text content. For a complete set of available effects, see the API documentation.
If you need to implement a text editing area in your JavaFX application, use the HTMLEditor
component. For more information about the HTMLEditor
control, see HTML Editor.