The list of alternate shipping addresses in the Address Book page provides a link to the Edit Shipping Address page, where the member can modify the address or its nickname.
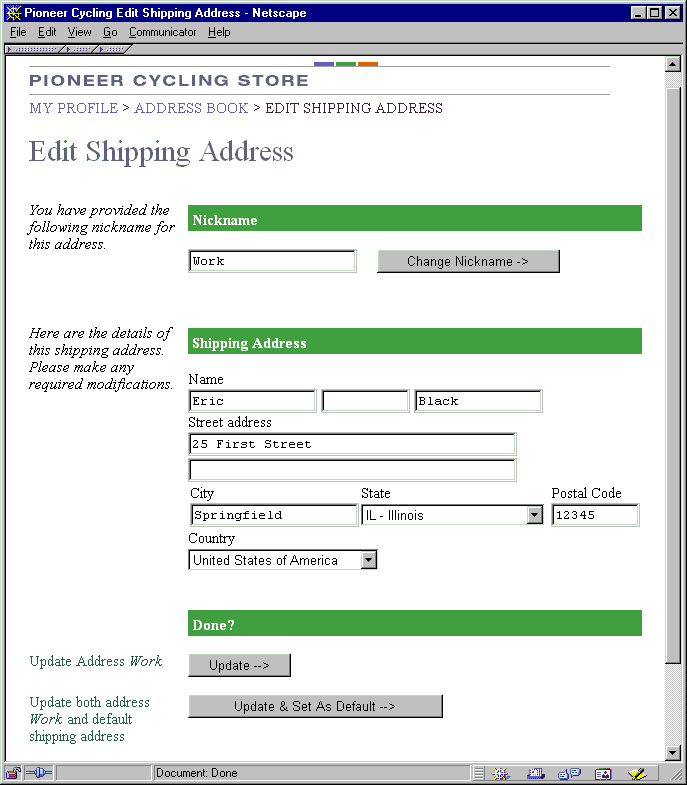
edit_shipping_address.jsp
There are two forms on this page. The first invokes handleChangeNickname()
to change the nickname of the address, the second updates the address data with changes entered by the user by invoking either handleUpdateAddress()
or handleUpdateAddressAndMakeDefault()
. The first only updates the address; the second updates the address and copies it to the default shipping address.
The operation of the upper form for changing the nickname of an address depends on two fields. One field is hidden and pre-populated with the old nickname of the address. The form handler uses this value to determine which address is being edited. The second form, which changes the contents of the address, also starts with the nickname in a hidden field for the same reason.
Because the B2CProfileFormHandler
is session scoped, you could create a property in the form handler in both cases and set it to the value of the address being edited when the user enters the form to edit the shipping address. There is no real benefit to one way or the other, but the way we implemented it in Pioneer Cycling would also work for a request scoped form handler.
Note: The “. . .” markers in the following code sample indicate a place where code has been removed to clarify the sample.
<dsp:form action="address_book.jsp" method="POST"> . . . <dsp:input bean="B2CProfileFormHandler.editValue.nickname" type="hidden"/> <dsp:input bean="B2CProfileFormHandler.editValue.newNickname" maxlength="40" size="20" type="text"/> <!-- Submit form to handleChangeAddressNickname() handler --> <dsp:input bean="B2CProfileFormHandler.changeAddressNickname" type="submit" value="Change Nickname ->"/> . . . </dsp:form> . . . <dsp:form action="address_book.jsp" method="POST"> . . . <dsp:input bean="B2CProfileFormHandler.editValue.nickname" type="hidden"/> Name<br> <dsp:input bean="B2CProfileFormHandler.editValue.firstName" maxlength="100" size="15" type="text" required="<%=true%>"/> . . . Street address <br> <dsp:input bean="B2CProfileFormHandler.editValue.address1" maxlength="30" size="40" type="text" required="<%=true%>"/><br> . . . <!-- Update Address --> <dsp:input bean="B2CProfileFormHandler.updateAddress" type="submit" value=" Update --"/> . . . <dsp:input bean="B2CProfileFormHandler.updateAddressAndMakeDefault" type="submit" value=" Update & Set As Default --"/> . . .
The editValue
property must be initialized to the current value of that shipping address before the Edit Shipping Address page is displayed to the member. The hyperlink in the Address Book page sets the editAddress
property of the ProfileFormHandler
to the ID of the address to be edited:
<dsp:a bean="B2CProfileFormHandler.editAddress" href="edit_shipping_address.jsp" paramvalue="key">edit this</dsp:a>
The property has an associated handler, handleEditAddress()
, that populates the editValue
property with the address data. It looks at the EditAddress
property for the ID of the address to edit:
String nickname = getEditAddress(); Map secondaryAddress = (Map) getProfile().getPropertyValue("secondaryAddresses"); MutableRepositoryItem theAddress = (MutableRepositoryItem) secondaryAddress.get(nickname); Map edit = getEditValue(); // save nickname in editValue map –- the updateAddress handler will need it edit.put("nickname", nickname); // ...as will, perhaps, the changeAddressNickname handler edit.put("newNickname", nickname); String[] addressProps = getAddressProperties(); // Store each property in the map, for use by edit_secondary_address.jsp Object property; for (int i = 0; i < addressProps.length; i++) { property = theAddress.getPropertyValue(addressProps[i]); if (property != null) edit.put(addressProps[i], property); }
The member makes any changes to the address and invokes one of the three submit buttons (Change Nickname, Update, or Update & Set As Default) to commit those changes.