In order to use the express checkout feature, the customer must have registered as a member, logged in as a member, and entered at least one valid credit card and one shipping address. Once these conditions are met, a customer may enable the express checkout feature by clicking see my express checkout ordering preferences under “My commerce profile” on the “My Profile” page and then clicking the Set Your Express Checkout Preferences button.
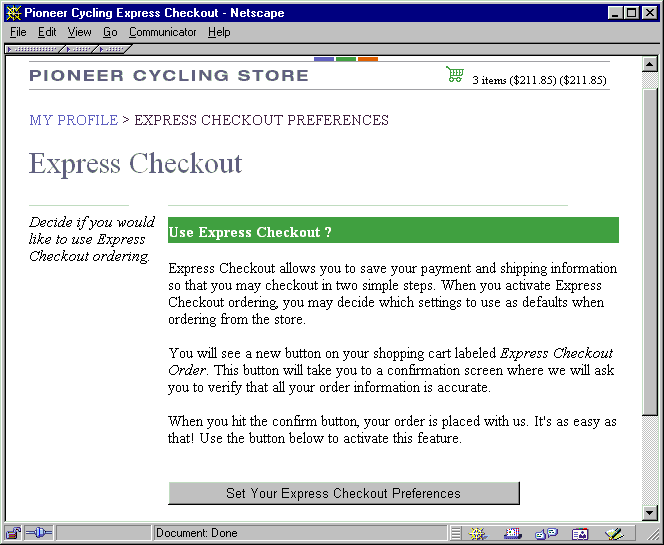
The hidden field in the form sample in express_checkout.jsp
below sets the form handler’s updateRepositoryId
property. This property is used by theB2CProfileFormHandler
to ensure that the profile being edited is in fact the profile that is associated with the current session (in case a session expires or otherwise becomes invalid while a person is filling in a form). We recommend using this hidden field in all forms that invoke the handleUpdate
method of ProfileFormHandler
or its subclasses.
<dsp:form action="express_checkout.jsp"> <dsp:input bean="B2CProfileFormHandler.updateRepositoryId" beanvalue="B2CProfileFormHandler.repositoryId" type="hidden"/>
After the user checks the Set Your Express Checkout Preferences button, she is presented with a form that asks for default shipping and billing information. The express checkout feature requires that at least one credit card already be entered. If the customer’s profile contains no credit cards, she is presented with a message and a link to the credit card entry page. After a customer has entered at least one credit card, she is presented again with the page above.
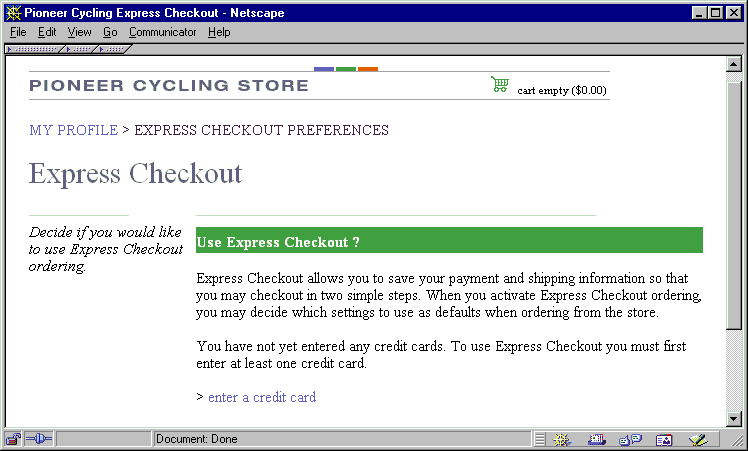
Users without a valid credit card see the message above
Users with valid credit cards see a form that lets them select a default credit card. Additionally, the “Save These Express Checkout Preferences” button is rendered.
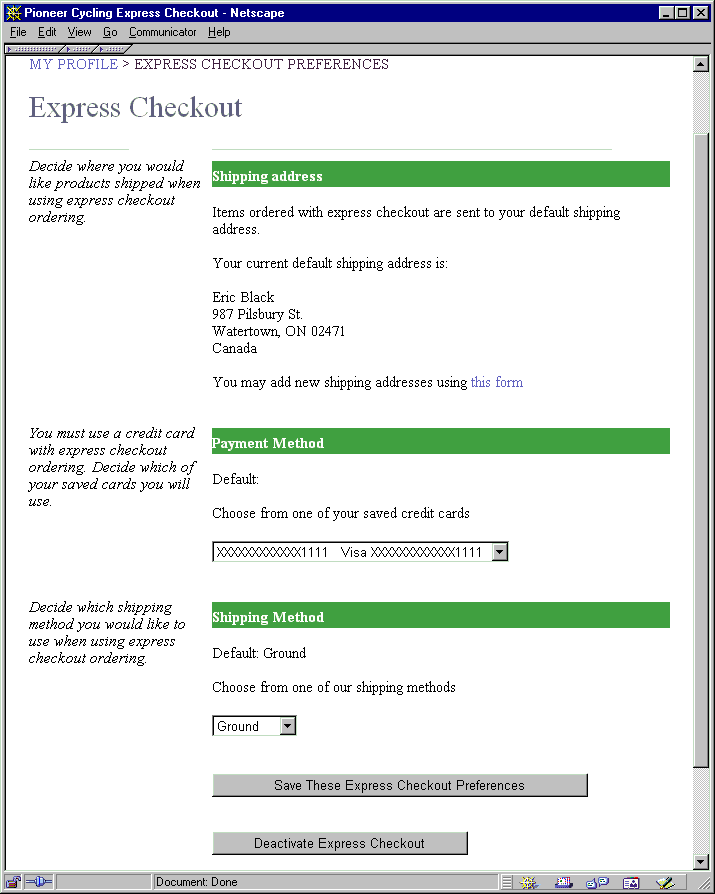
Setting Express Checkout preferences
When the customer pushes the Save These Express Checkout Preferences button, the expressCheckout
property of the user’s profile is set. This ensures that the address, credit card, and shipping methods have been set and prevents invalid data from causing errors at checkout time.
Setting the Express Checkout preferences is specialized Pioneer Cycling Store functionality. This code can be found in <ATG2007.3dir>/PioneerCyclingJSP/j2ee-apps/pioneer/web-app/en/user/express_checkout_preferences.jsp
.
The first form element on the page is the pull-down list for selecting the default shipping address. The customer’s default shipping address is stored in the property Profile.shippingAddress
. The pull-down list in the screen shot above shows the list of all nicknames of addresses stored in Profile.secondaryAddressess
. The pull down list is linked to the property ProfileFormHandler.editValue.defaultAddressNickname
. When the form is submitted, the selected value is set in the Profile.shippingAddress
property. The following snippet demonstrates how the shipping address is set.
Your current default shipping address is: <!-- <b><dsp:valueof param="key"/></b>. --> <p> <dsp:getvalueof id="pval0" bean="Profile.shippingAddress"><dsp:include page="DisplayAddress.jsp" flush="true"><dsp:param name="address" value="<%=pval0%>"/></dsp:include></dsp:getvalueof> <p> <dsp:droplet name="IsEmpty"> <dsp:param bean="Profile.secondaryAddresses" name="value"/> <dsp:oparam name="false"> Change it to: <dsp:select bean="B2CProfileFormHandler.editValue.defaultAddressNickname"> <dsp:droplet name="ForEach"> <dsp:param bean="Profile.secondaryAddresses" name="array"/> <dsp:oparam name="outputStart"> <dsp:option value=""/> Don't Change </dsp:oparam> <dsp:oparam name="output"> <dsp:droplet name="Switch"> <dsp:param name="value" param="key"/> <dsp:oparam name="editAddress"> </dsp:oparam> <dsp:oparam name="newBillingAddress"> </dsp:oparam> <dsp:oparam name="default"> <dsp:getvalueof id="option119" param="key" idtype="java.lang.String"> <dsp:option value="<%=option119%>"/> </dsp:getvalueof><dsp:valueof param="key"/> </dsp:oparam> </dsp:droplet> </dsp:oparam> </dsp:droplet> </dsp:select>
The concepts involved in setting the default credit card are similar to setting the shipping address. The default credit card is stored in Profile.defaultCreditCard
, which is a reference to a RepositoryItem
of type credit_card
that has the properties creditCardType
and creditCardNumber
. These are displayed on the page next to the word “Default:” to remind the user of the current setting, if any. Just below that is a pull-down list for selecting a new default credit card that shows a list of all credit cards on the user’s profile.
To display the current default value as pre-selected, we used the <dsp:setvalue>
to set the form handler’s value for the default credit card from the profile. To actually render the <dsp:select>
drop-down list, we used an IsEmpty
servlet bean to determine if there is anything to render at all. The ForEach
servlet bean has an empty oparam
that one might expect to be able to use for the case when the credit cards are empty and an oparam="outputStart"
for the <dsp:select>
tag and oparam="outputEnd"
for the </dsp:select>
. The ForEach
servlet bean has been tuned for top performance and it would be too inefficient for the page compiler to figure out which <dsp:select>
and </dsp:select>
and <dsp:option>
tags go together. If we used a loosely coupled group, like radio buttons, the IsEmpty
would not be necessary, but since we used select
, we must help the page compiler by using IsEmpty
.
Default: <dsp:valueof bean="Profile.defaultCreditCard.creditCardType"/> <dsp:valueof bean="Profile.defaultCreditCard.creditCardNumber" converter="creditcard"/> <p> <dsp:setvalue bean="B2CProfileFormHandler.defaultCreditCardID" beanvalue="Profile.defaultCreditCard.id"/> <dsp:droplet name="IsEmpty"> <dsp:param bean="Profile.creditCards" name="value"/> <dsp:oparam name="true"> <dsp:a href="credit_cards.jsp"> <font color=#cc0000> Please enter one or more credit cards in order to enable this feature </font> </dsp:a> <br> </dsp:oparam> <dsp:oparam name="false"> Choose from one of your saved credit cards<p> <dsp:select bean="B2CProfileFormHandler.defaultCreditCardID"> <dsp:droplet name="ForEach"> <dsp:param bean="Profile.creditCards" name="array"/> <dsp:oparam name="output"> <dsp:getvalueof id="option226" param="element.id" idtype="java.lang.String"> <dsp:option value="<%=option226%>"/> </dsp:getvalueof> <dsp:valueof converter="creditcard" param="key"/> <dsp:valueof param="element.creditCardType"/> <dsp:valueof converter="creditcard" param="element.creditCardNumber"/> </dsp:oparam> </dsp:droplet> </dsp:select> </dsp:oparam> </dsp:droplet>
While credit cards and shipping addresses that must be drawn from the user’s profile, setting the shipping method is simpler because the list of possible shipping methods is common to all users. We used the same methods as above to pre-set the selected item on the pull-down list of shipping methods. The actual pull down list is generated by the AvailableShippingMethods
servlet bean that is described in the Shipping Prices section of the Pricing chapter.
Default: <dsp:valueof bean="Profile.defaultCarrier"/> <p> <dsp:setvalue bean="B2CProfileFormHandler.defaultCarrier" beanvalue="Profile.defaultCarrier"/> Choose from one of our shipping methods<p> <!-- The droplet AvailableShippingMethods is able to give us a list of the --> <!-- shipping methods that should be available to a user. --> <dsp:droplet name="AvailableShippingMethods"> <dsp:param bean="ShoppingCartModifier.shippingGroup" name="shippingGroup"/> <dsp:param bean="UserPricingModels.shippingPricingModels" name="pricingModels"/> <dsp:param bean="Profile" name="profile"/> <dsp:oparam name="output"> <dsp:select bean="B2CProfileFormHandler.defaultCarrier"> <dsp:droplet name="ForEach"> <dsp:param name="array" param="availableShippingMethods"/> <dsp:param name="elementName" value="method"/> <dsp:oparam name="output"> <dsp:getvalueof id="option313" param="method" idtype="java.lang.String"> <dsp:option value="<%=option313%>"/> </dsp:getvalueof> <dsp:valueof param="method"/> </dsp:oparam> </dsp:droplet> </dsp:select> </dsp:oparam> </dsp:droplet>
The last form element on the page is the Save These Express Checkout Preferences submit button. This button is rendered only if the list of credit cards in the profile is not empty. The form is submitted to B2CProfileFormHandler.setExpressCheckoutPreferences
, which takes the values from the form fields and saves them to the profile.
<dsp:droplet name="IsEmpty"> <dsp:param bean="Profile.creditCards" name="value"/> <dsp:oparam name="false"> <dsp:input bean="B2CProfileFormHandler.setExpressCheckoutPreferences" type="submit" value="Save These Express Checkout Preferences"/> </dsp:oparam> </dsp:droplet>