For each SKU of the product on the template page, we wanted to display some inventory information: SKU display name, price, and inventory level.
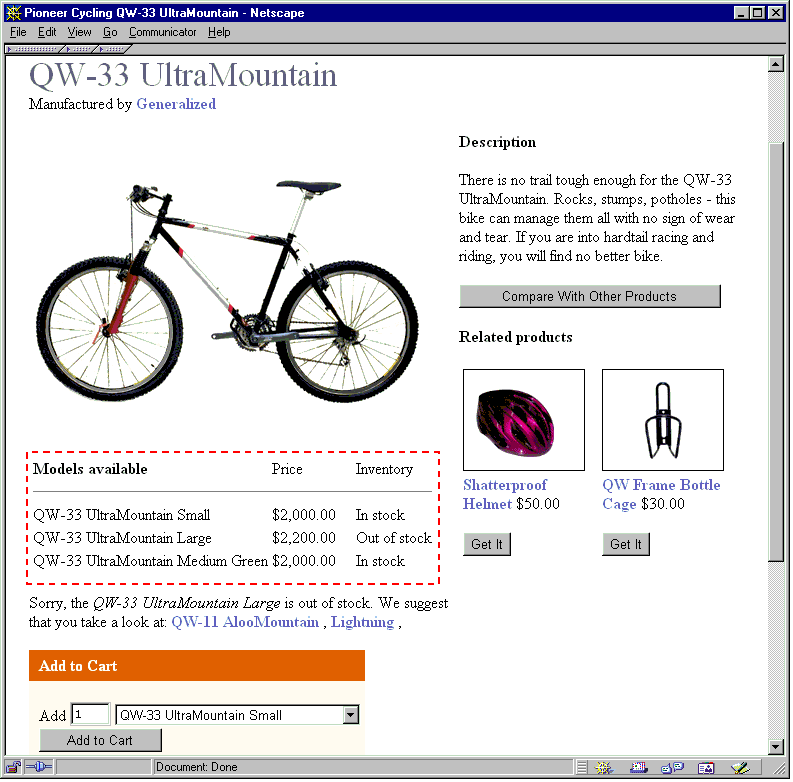
Inventory Information
We needed this functionality on all of our template pages so we put the code into a separate code fragment called DisplaySkuInfo.jsp
.
Here is an example of how this code fragment is included from the template page. The code fragment is passed the Product
parameter whose SKUs need to be displayed:
<dsp:getvalueof id="pval0" param="Product"> <dsp:include page="common/DisplaySkuInfo.jsp" flush="true"> <dsp:param name="Product" value="<%=pval0%>"/></dsp:include></dsp:getvalueof>
This is the actual code for the DisplaySkuInfo.jsp
code fragment:
<DECLAREPARAM NAME="Product" CLASS="java.lang.Object" DESCRIPTION="A Product repository Item - REQUIRED."> <dsp:importbean bean="/atg/commerce/pricing/PriceItem"/> <dsp:importbean bean="/atg/commerce/inventory/InventoryLookup"/> <dsp:droplet name="/atg/dynamo/droplet/ForEach"> <dsp:param name="array" param="Product.childSKUs"/> <dsp:param name="elementName" value="sku"/> <dsp:oparam name="outputStart"> <table cellspacing=4 cellpadding=0 border=0> <tr> <td> <b>Models available</b> </td> <td> Price </td> <td> </td> <td> Inventory </td> </tr> <tr><td colspan=4><hr size=0></td></tr> </dsp:oparam> <dsp:oparam name="outputEnd"> </table> </dsp:oparam> <dsp:oparam name="output"> <dsp:droplet name="InventoryLookup"> <dsp:param name="itemId" param="sku.repositoryId"/> <dsp:param name="useCache" value="true"/> <dsp:oparam name="output"> <tr valign=top> <td><dsp:valueof param="sku.displayName"/></td> <td> <dsp:getvalueof id="pval0" param="sku"> <dsp:include page="../../common/DisplaySkuPrice.jsp" flush="true"> <dsp:param name="Sku" value="<%=pval0%>"/> </dsp:include></dsp:getvalueof><br> </td> <td></td> <td> <dsp:valueof param="inventoryInfo.availabilityStatusMsg">Unknown </dsp:valueof></td> </tr> </dsp:oparam> </dsp:droplet> </dsp:oparam> </dsp:droplet> <%/*ForEach sku droplet*/%>
To display information about each of the child SKUs of a product, we used the ForEach
component. ForEach
is passed an array that is the list of SKUs associated with the product (the childSkus
property value). As the code cycles through each of the SKUs in the product, it binds the Sku
parameter to the current SKU. Here is the start of this code section:
<dsp:droplet name="/atg/dynamo/droplet/ForEach"> <dsp:param name="array" param="Product.childSKUs"/> <dsp:param name="elementName" value="sku"/> . . .
The Sku
parameter that was bound in the ForEach
component is used to access property values on the SKU. Any information to be displayed on the page is put in the output
parameter sections of ForEach
(for example, output
, outputStart
, or outputEnd
). To display the SKU’s displayName
attribute, we did the following:
<dsp:droplet name="/atg/dynamo/droplet/ForEach"> <dsp:param name="array" param="Product.childSKUs"/> <dsp:param name="elementName" value="sku"/> . . . <dsp:oparam name="output"> . . . <dsp:valueof param="sku.displayName"/> . . . </dsp:oparam> </dsp:droplet>
We used the DisplaySkuPrice.jsp
code fragment to display the SKU’s price.
<dsp:getvalueof id=""pval10" param="sku"> <dsp:include page="../../common/DisplaySkuPrice.jsp" flush="true"> <dsp:param name="Sku" value="<%=pval0%>"/> </dsp:include> </dsp:getvalueof>
We used the InventoryLookup
component (which is explained in more detail later) to display the SKU’s inventory level:
<dsp:droplet name="InventoryLookup"> <dsp:param name="itemId" param="sku.repositoryId"/> <dsp:param name="useCache" value="true"/> <dsp:oparam name="output"> <dsp:valueof param="sku.displayName"/> <dsp:getvalueof id="pval0" param="sku"> <dsp:include page="../../common/DisplaySkuPrice.jsp" flush="true"> <dsp:param name="Sku" value="<%=pval0%>"/> </dsp:include> </dsp:getvalueof><br> <dsp:valueof param="inventoryInfo.availabilityStatusMsg">Unknown </dsp:valueof> </dsp:oparam> </dsp:droplet>